用Fortran90的subroutine用二分法求解非线性方程
时间: 2024-02-28 15:56:47 浏览: 114
好的,下面是一个用Fortran90编写的求解非线性方程的二分法子程序的示例代码:
```
subroutine bisection(f, a, b, x, tol, maxiter)
! 定义输入参数
real*8, external :: f
real*8, intent(in) :: a, b, tol
integer, intent(in) :: maxiter
! 定义输出参数
real*8, intent(out) :: x
! 定义局部变量
real*8 :: fa, fb, fx, xm
integer :: iter
! 初始值
fa = f(a)
fb = f(b)
if (fa*fb > 0.0d0) then
print*, "Error: f(a) and f(b) must have opposite signs."
return
end if
xm = (a + b) / 2.0d0
fx = f(xm)
iter = 0
! 迭代求解
do while (abs(fx) > tol .and. iter < maxiter)
if (fa*fx < 0.0d0) then
b = xm
fb = fx
else
a = xm
fa = fx
end if
xm = (a + b) / 2.0d0
fx = f(xm)
iter = iter + 1
end do
! 输出结果
x = xm
if (iter == maxiter) then
print*, "Warning: maximum number of iterations reached."
end if
end subroutine bisection
```
其中,`f`是一个外部定义的函数,输入一个实数$x$,输出$f(x)$的值。`a`和`b`是初始的搜索区间,`tol`是收敛精度,`maxiter`是最大迭代次数。`x`是输出结果,即非线性方程的解。
您可以将上述代码保存为一个Fortran90源文件,然后在您的主程序中调用`bisection`子程序来求解非线性方程。例如:
```
program main
implicit none
real*8 :: a, b, x, tol
integer :: maxiter
! 设置参数
a = 0.0d0
b = 1.0d0
tol = 1.0d-6
maxiter = 100
! 调用bisection子程序
call bisection(f, a, b, x, tol, maxiter)
! 输出结果
print*, "The solution is: x =", x
contains
function f(x)
real*8 :: x, f
f = x**3 - x**2 + 2.0d0*x - 1.0d0
end function f
end program main
```
其中,`f`函数定义了要求解的非线性方程,本例中为$x^3 - x^2 + 2x - 1 = 0$。主程序中设置了参数,调用`bisection`子程序求解方程,并输出结果。
阅读全文
相关推荐









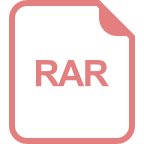




