train_data,test_data,train_label,test_label = model_selection.train_test_split(x,y, random_state=1, train_size=0.9,test_size=0.1)
时间: 2023-07-24 12:15:32 浏览: 155
这是一个用于数据集划分的代码,将数据集按照一定比例划分为训练集和测试集。其中,x为特征矩阵,y为标签矩阵。train_size和test_size分别指定了训练集和测试集所占的比例。random_state为随机种子,用于确保每次划分的结果都相同。具体来说,这段代码将数据集划分为90%的训练集和10%的测试集,并将划分好的数据分别赋值给train_data, test_data, train_label, test_label。
相关问题
import pandas as pd import numpy as np from sklearn.model_selection import train_test_split pd.set_option('display.max_columns', None) # 所有列 pd.set_option('display.max_rows', None) # 所有行 data = pd.read_excel('半监督数据.xlsx') X = data.drop(columns=['label']) # 特征矩阵 y = data['label'] # 标签列 # 划分数据集 X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.1, stratify=None, shuffle=True, random_state=0) # 划分带标签数据集 labeled_size = 0.3 n_labeled = int(labeled_size * len(X_train)) indices = np.arange(len(X_train)) unlabeled_indices = np.delete(indices, y_train.index[:n_labeled]) X_unlabeled = X_train.iloc[unlabeled_indices] y_unlabeled = y_train.iloc[unlabeled_indices] X_labeled = X_train.iloc[y_train.index[:n_labeled]] y_labeled = y_train.iloc[y_train.index[:n_labeled]] from sklearn import preprocessing pre_transform=preprocessing.StandardScaler() pre_transform.fit(np.vstack([train_datas, test_datas])) train_datas=pre_transform.transform(train_datas) test_datas=pre_transform.transform(train_datas) from LAMDA_SSL.Algorithm.Regression.CoReg import CoReg model=CoReg() model.fit(X=train_datas,y=labeled_y,test_datas=unlabeled_X) pred_y=model.predict(X=test_X) from LAMDA_SSL.Evaluation.Regressor.Mean_Squared_Error import Mean_Squared_Error performance = Mean_Squared_Error().scoring(test_y, pred_y)帮我看一下这段代码有什么问题?怎么修改?
在代码中,预处理部分中使用了未定义的变量 `train_datas` 和 `test_datas`,应该将其改为 `X_train` 和 `X_test`。
另外,在调用 `CoReg` 模型时,传入的参数名 `test_datas` 应该改为 `unlabeled_X`,因为在之前的代码中,`X_unlabeled` 被赋值给了 `unlabeled_X`。
最后,在计算预测结果时,应该将 `test_X` 改为 `X_test`。
修改后的代码如下:
```
import pandas as pd
import numpy as np
from sklearn.model_selection import train_test_split
from sklearn import preprocessing
from LAMDA_SSL.Algorithm.Regression.CoReg import CoReg
from LAMDA_SSL.Evaluation.Regressor.Mean_Squared_Error import Mean_Squared_Error
pd.set_option('display.max_columns', None) # 所有列
pd.set_option('display.max_rows', None) # 所有行
data = pd.read_excel('半监督数据.xlsx')
X = data.drop(columns=['label']) # 特征矩阵
y = data['label'] # 标签列
# 划分数据集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.1, stratify=None, shuffle=True, random_state=0)
# 划分带标签数据集
labeled_size = 0.3
n_labeled = int(labeled_size * len(X_train))
indices = np.arange(len(X_train))
unlabeled_indices = np.delete(indices, y_train.index[:n_labeled])
X_unlabeled = X_train.iloc[unlabeled_indices]
y_unlabeled = y_train.iloc[unlabeled_indices]
X_labeled = X_train.iloc[y_train.index[:n_labeled]]
y_labeled = y_train.iloc[y_train.index[:n_labeled]]
# 数据预处理
pre_transform=preprocessing.StandardScaler()
pre_transform.fit(np.vstack([X_train, X_test]))
X_train = pre_transform.transform(X_train)
X_test = pre_transform.transform(X_test)
# 构建和训练模型
model = CoReg()
model.fit(X=X_train, y=y_labeled, test_datas=X_unlabeled)
pred_y = model.predict(X=X_test)
# 计算性能指标
performance = Mean_Squared_Error().scoring(y_test, pred_y)
```
import pandas as pd import matplotlib.pyplot as plt import seaborn as sns from sklearn.model_selection import train_test_split from sklearn.preprocessing import StandardScaler from sklearn.ensemble import RandomForestClassifier from sklearn.metrics import confusion_matrix, classification_report, accuracy_score # 1. 数据准备 train_data = pd.read_csv('train.csv') test_data = pd.read_csv('test_noLabel.csv') # 填充缺失值 train_data.fillna(train_data.mean(), inplace=True) test_data.fillna(test_data.mean(), inplace=True) # 2. 特征工程 X_train = train_data.drop(['Label', 'ID'], axis=1) y_train = train_data['Label'] X_test = test_data.drop('ID', axis=1) scaler = StandardScaler() X_train = scaler.fit_transform(X_train) X_test = scaler.transform(X_test) # 3. 模型建立 model = RandomForestClassifier(n_estimators=100, random_state=42) # 4. 模型训练 model.fit(X_train, y_train) # 5. 进行预测 y_pred = model.predict(X_test) # 6. 保存预测结果 df_result = pd.DataFrame({'ID': test_data['ID'], 'Label': y_pred}) df_result.to_csv('forecast_result.csv', index=False) # 7. 模型评估 y_train_pred = model.predict(X_train) print('训练集准确率:', accuracy_score(y_train, y_train_pred)) print('测试集准确率:', accuracy_score(y_test, y_pred)) print(classification_report(y_test, y_pred)) # 8. 绘制柱形图 feature_importances = pd.Series(model.feature_importances_, index=X_train.columns) feature_importances = feature_importances.sort_values(ascending=False) plt.figure(figsize=(10, 6)) sns.barplot(x=feature_importances, y=feature_importances.index) plt.xlabel('Feature Importance Score') plt.ylabel('Features') plt.title('Visualizing Important Features') plt.show() # 9. 对比类分析 train_data['Label'].value_counts().plot(kind='bar', color=['blue', 'red']) plt.title('Class Distribution') plt.xlabel('Class') plt.ylabel('Frequency') plt.show()
这段代码是一个机器学习模型的完整流程,包括数据准备、特征工程、模型建立、模型训练、预测结果保存、模型评估和可视化分析等步骤。其中包括了绘制柱形图和对比类分析的代码。
绘制柱形图的代码如下:
```
feature_importances = pd.Series(model.feature_importances_, index=X_train.columns)
feature_importances = feature_importances.sort_values(ascending=False)
plt.figure(figsize=(10, 6))
sns.barplot(x=feature_importances, y=feature_importances.index)
plt.xlabel('Feature Importance Score')
plt.ylabel('Features')
plt.title('Visualizing Important Features')
plt.show()
```
这段代码会生成一个柱形图,横轴为特征重要性得分,纵轴为特征名称,用于展示机器学习模型中各个特征的重要性。
对比类分析的代码如下:
```
train_data['Label'].value_counts().plot(kind='bar', color=['blue', 'red'])
plt.title('Class Distribution')
plt.xlabel('Class')
plt.ylabel('Frequency')
plt.show()
```
这段代码会生成一个柱形图,展示训练数据中不同类别的样本数量分布情况。这个图可以用于判断数据集是否存在类别不平衡的情况,以及对模型的训练和评估结果进行解释。
阅读全文
相关推荐
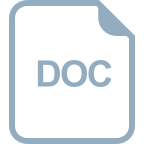
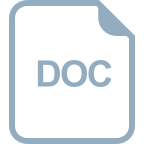
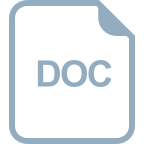














