#include<iostream> using namespace std; class Point { private: int x,y; public: Point(int x=0,int y=0):x(x),y(y){};}; class Rectangle { private: Point p1,p2; int length, width,perimeter,area; public: Rectangle(Point p1,Point p2):p1(p1),p2(p2){ int width=int(p2.Point(x)-p1.Point(x)); int length=int(p2.Point(y)-p1.Point(y)); perimeter=2*(width+length); area=width*length; } int getPerimeter(){return perimeter;} int getArea(){return area;} }; int main() { Point p1(1,1); Point p2(2,2); Rectangle rect(p1,p2); cout<<"周长为"<<rect.getPerimeter()<<endl; cout<<"面积为"<<rect.getArea()<<endl; return 0; }
时间: 2023-08-21 17:49:57 浏览: 97
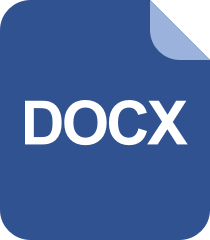
实验1 VC 简单程序设计.docx
这是一个 C++ 程序,定义了两个类 Point 和 Rectangle。Point 类表示平面上的一个点,Rectangle 类表示矩形。在 main 函数中创建了两个 Point 对象 p1 和 p2,然后通过这两个点创建了一个 Rectangle 对象 rect。程序计算了矩形的周长和面积,并输出到控制台。
阅读全文
相关推荐
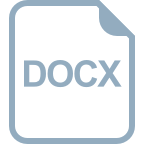
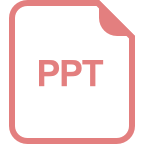














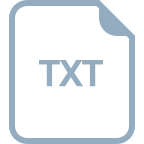
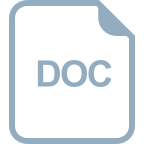