如何根据MPU6050的俯仰角,反转角,航向角控制物体
时间: 2023-06-02 20:03:12 浏览: 66
根据MPU6050的俯仰角、反转角和航向角控制物体的方法取决于具体的应用场景和需求。以下是一些常见的方法:
1. 控制飞行器姿态:通过读取MPU6050的俯仰角、反转角和航向角,可以控制飞行器的姿态,使其保持水平或者进行特定的姿态调整。
2. 控制机器人运动:可以使用MPU6050的角度数据来控制机器人的运动,例如让机器人沿着一条特定的路径移动或者绕过障碍物。
3. 控制摄像头方向:可以使用MPU6050的角度数据来控制摄像头的方向,例如让摄像头跟随物体移动或者保持固定的方向。
4. 控制游戏角色移动:可以使用MPU6050的角度数据来控制游戏角色的移动,例如让角色跳跃或者向左/右移动。
需要注意的是,以上方法都需要将MPU6050的角度数据与具体的控制算法相结合,才能实现有效的控制效果。同时,不同的物体控制方法也需要针对具体的硬件平台和软件环境进行适配和优化。
相关问题
如何根据MPU6050的俯仰角,反转角,航向角控制游戏角色移动
MPU6050是一个六轴传感器,能够测量俯仰角、反转角和航向角。根据这些测量值,可以使用Arduino或其他微控制器控制游戏角色的移动。以下是一个简单的示例程序,使用MPU6050控制游戏角色在平面上移动。
首先,需要连接MPU6050到Arduino。可以使用I2C接口连接,具体连接方式可以参考MPU6050的数据手册。然后,需要安装MPU6050库,可以在Arduino IDE的库管理器中找到。
接下来,需要编写程序来读取MPU6050的测量值,并将其转换为游戏角色的移动指令。可以使用以下代码:
```
#include <Wire.h>
#include <MPU6050.h>
MPU6050 mpu;
void setup() {
Wire.begin();
mpu.initialize();
}
void loop() {
float pitch, roll, yaw;
mpu.getMotion6(&ax, &ay, &az, &gx, &gy, &gz);
pitch = atan2(ax, sqrt(ay * ay + az * az)) * 180 / PI;
roll = atan2(ay, sqrt(ax * ax + az * az)) * 180 / PI;
yaw = atan2(sqrt(ax * ax + ay * ay), az) * 180 / PI;
//根据pitch, roll, yaw计算游戏角色的移动指令
// ...
delay(10);
}
```
在这个代码中,使用MPU6050库中的getMotion6函数读取MPU6050的六轴测量值。然后,使用三角函数计算俯仰角、反转角和航向角。
接下来,根据测量值计算游戏角色的移动指令。例如,可以使用俯仰角和反转角控制游戏角色的左右移动和上下移动。可以使用航向角控制游戏角色的旋转。
最后,需要将计算出来的移动指令发送到游戏中。可以使用串口或其他通信方式将指令发送到游戏中。
需要注意的是,这个示例程序只是一个简单的示例,具体的移动指令和控制方式需要根据实际情况进行调整。同时,需要根据游戏的要求进行优化,以确保游戏角色的移动流畅和精准。
MPU6050输出俯仰角,反转角,航向角控制UNITY小球运动
要实现MPU6050输出俯仰角,反转角和航向角控制UNITY小球运动,需要进行以下步骤:
1. 连接MPU6050传感器和Arduino板。
2. 使用Arduino IDE编写代码,读取MPU6050的数据并计算俯仰角,反转角和航向角。
3. 将计算得到的角度数据通过串口传输到Unity中。
4. 在Unity中创建小球模型,并编写脚本,接收通过串口传输的角度数据,控制小球的运动。
以下是示例代码:
Arduino代码:
```
#include <Wire.h>
#include <MPU6050.h>
MPU6050 mpu;
void setup() {
Serial.begin(9600);
Wire.begin();
mpu.initialize();
}
void loop() {
float ax, ay, az;
float gx, gy, gz;
mpu.getMotion6(&ax, &ay, &az, &gx, &gy, &gz);
float roll = atan2(ay, az) * 180 / PI;
float pitch = atan2(-ax, sqrt(ay * ay + az * az)) * 180 / PI;
float yaw = atan2((gz * sin(pitch) - gy * cos(pitch)), (gx * cos(roll) + gy * sin(roll) * sin(pitch) + gz * sin(roll) * cos(pitch))) * 180 / PI;
Serial.print("Roll: ");
Serial.print(roll);
Serial.print(" Pitch: ");
Serial.print(pitch);
Serial.print(" Yaw: ");
Serial.println(yaw);
delay(100);
}
```
Unity代码:
```
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using System.IO.Ports;
public class MPU6050Control : MonoBehaviour {
SerialPort sp = new SerialPort("COM3", 9600);
void Start() {
sp.Open();
sp.ReadTimeout = 1;
}
void Update() {
if (sp.IsOpen) {
try {
string data = sp.ReadLine();
string[] angles = data.Split(' ');
float roll = float.Parse(angles[1]);
float pitch = float.Parse(angles[3]);
float yaw = float.Parse(angles[5]);
Vector3 rotation = new Vector3(-pitch, yaw, roll);
transform.rotation = Quaternion.Euler(rotation);
} catch (System.Exception) {
}
}
}
void OnApplicationQuit() {
sp.Close();
}
}
```
在Unity中创建一个空对象,将上述代码添加到该对象上,然后将小球模型作为其子对象,并将脚本拖到空对象上。
通过上述步骤,就可以实现MPU6050输出俯仰角,反转角和航向角控制UNITY小球运动。
相关推荐
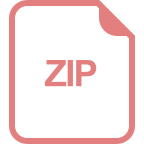
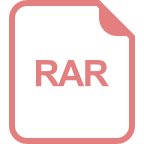












