八邻域 python 巡线
时间: 2023-09-13 07:07:08 浏览: 104
如果您想使用八邻域来进行巡线,您可以使用OpenCV和Python来实现。下面是一个示例代码,展示了如何使用八邻域进行巡线:
```python
import cv2
import numpy as np
# 读取图像
image = cv2.imread("line_image.jpg")
# 转换为灰度图像
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# 二值化图像
ret, binary = cv2.threshold(gray, 127, 255, cv2.THRESH_BINARY)
# 提取边缘
edges = cv2.Canny(binary,50, 150)
# 获取图像尺寸
height, width = edges.shape
# 定义八个方向的像素位移
dx = [-1, -1, 0, 1, 1, 1, 0, -1]
dy = [0, 1, 1, 1, 0, -1, -1, -1]
# 初始化起始位置
start_x = int(width / 2)
start_y = height - 1
# 巡线过程
current_x = start_x
current_y = start_y
path = [(current_x, current_y)]
while current_y > 0:
max_intensity = 0
next_x = current_x
next_y = current_y
for i in range(8):
new_x = current_x + dx[i]
new_y = current_y + dy[i]
if new_x >= 0 and new_x < width and new_y >= 0 and new_y < height:
intensity = edges[new_y, new_x]
if intensity > max_intensity:
max_intensity = intensity
next_x = new_x
next_y = new_y
current_x = next_x
current_y = next_y
path.append((current_x, current_y))
# 绘制巡线路径
for point in path:
cv2.circle(image, point, 1, (0, 0, 255), -1)
# 显示结果
cv2.imshow("Line Following", image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
在上述代码中,我们首先读取图像并将其转换为灰度图像。然后,对图像进行二值化处理,并使用Canny边缘检测算法提取边缘。接下来,我们定义了八个方向的像素位移,并初始化起始位置为图像底部中间位置。然后,我们通过选择具有最大边缘强度的相邻像素来进行巡线,并将路径上的像素保存在`path`列表中。最后,我们将巡线路径绘制在原始图像上,并显示结果。
请注意,上述代码仅是一个简单的示例,您可能需要根据您的实际应用进行适当的调整和优化。
希望这可以帮助到您!如果您有任何进一步的问题,请随时提问。
相关推荐
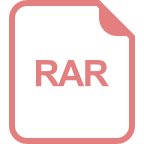
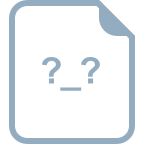














