进程哲学家进餐问题c代码
时间: 2024-06-13 08:09:34 浏览: 13
以下是进程实现哲学家进餐问题的C代码,其中使用了信号量来解决死锁问题,并且在控制台输出每个哲学家的工作状态:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <pthread.h>
#include <semaphore.h>
#define N 5 // 哲学家数量
#define LEFT (i + N - 1) % N // 左邻居编号
#define RIGHT (i + 1) % N // 右邻居编号
#define THINKING 0 // 思考状态
#define HUNGRY 1 // 饥饿状态
#define EATING 2 // 就餐状态
int state[N]; // 哲学家状态数组
sem_t mutex; // 互斥信号量
sem_t s[N]; // 哲学家信号量
void test(int i) {
if (state[i] == HUNGRY && state[LEFT] != EATING && state[RIGHT] != EATING) {
state[i] = EATING;
printf("哲学家 %d 正在就餐\n", i);
sem_post(&s[i]);
}
}
void take_forks(int i) {
sem_wait(&mutex);
state[i] = HUNGRY;
printf("哲学家 %d 饿了\n", i);
test(i);
sem_post(&mutex);
sem_wait(&s[i]);
sleep(1);
}
void put_forks(int i) {
sem_wait(&mutex);
state[i] = THINKING;
printf("哲学家 %d 放下了叉子\n", i);
test(LEFT);
test(RIGHT);
sem_post(&mutex);
}
void *philosopher(void *arg) {
int i = *(int *)arg;
while (1) {
printf("哲学家 %d 正在思考\n", i);
sleep(1);
take_forks(i);
put_forks(i);
}
}
int main() {
pthread_t tid[N];
int i, num[N];
sem_init(&mutex, 0, 1);
for (i = 0; i < N; i++) {
sem_init(&s[i], 0, 0);
num[i] = i;
state[i] = THINKING;
}
for (i = 0; i < N; i++) {
pthread_create(&tid[i], NULL, philosopher, &num[i]);
}
for (i = 0; i < N; i++) {
pthread_join(tid[i], NULL);
}
return 0;
}
```
相关推荐
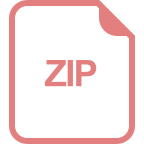
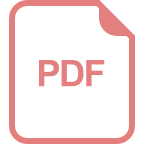
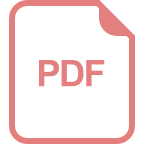














