帮我用c语言实现一个线程池
时间: 2023-11-23 13:49:24 浏览: 30
当然可以帮你实现一个简单的线程池。下面是一个用C语言实现的线程池的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#define MAX_THREADS 5
#define MAX_TASKS 10
typedef struct {
void (*function)(void* arg);
void* arg;
} task_t;
typedef struct {
task_t* tasks;
int front;
int rear;
int count;
int size;
pthread_mutex_t lock;
pthread_cond_t notify_producer;
pthread_cond_t notify_consumer;
} threadpool_t;
void threadpool_init(threadpool_t* pool, int size);
void threadpool_destroy(threadpool_t* pool);
void threadpool_add_task(threadpool_t* pool, void (*function)(void* arg), void* arg);
void threadpool_run(threadpool_t* pool);
void* threadpool_worker(void* arg);
void task_function(void* arg);
void threadpool_init(threadpool_t* pool, int size) {
pool->tasks = (task_t*)malloc(sizeof(task_t) * MAX_TASKS);
pool->front = 0;
pool->rear = 0;
pool->count = 0;
pool->size = size;
pthread_mutex_init(&(pool->lock), NULL);
pthread_cond_init(&(pool->notify_producer), NULL);
pthread_cond_init(&(pool->notify_consumer), NULL);
for (int i = 0; i < size; i++) {
pthread_t tid;
pthread_create(&tid, NULL, threadpool_worker, (void*)pool);
}
}
void threadpool_destroy(threadpool_t* pool) {
free(pool->tasks);
pthread_mutex_destroy(&(pool->lock));
pthread_cond_destroy(&(pool->notify_producer));
pthread_cond_destroy(&(pool->notify_consumer));
}
void threadpool_add_task(threadpool_t* pool, void (*function)(void* arg), void* arg) {
pthread_mutex_lock(&(pool->lock));
while (pool->count == MAX_TASKS) {
pthread_cond_wait(&(pool->notify_producer), &(pool->lock));
}
pool->tasks[pool->rear].function = function;
pool->tasks[pool->rear].arg = arg;
pool->rear = (pool->rear + 1) % MAX_TASKS;
pool->count++;
pthread_cond_signal(&(pool->notify_consumer));
pthread_mutex_unlock(&(pool->lock));
}
void threadpool_run(threadpool_t* pool) {
for (int i = 0; i < MAX_TASKS; i++) {
threadpool_add_task(pool, task_function, (void*)(intptr_t)i);
}
}
void* threadpool_worker(void* arg) {
threadpool_t* pool = (threadpool_t*)arg;
while (1) {
pthread_mutex_lock(&(pool->lock));
while (pool->count == 0) {
pthread_cond_wait(&(pool->notify_consumer), &(pool->lock));
}
task_t task = pool->tasks[pool->front];
pool->front = (pool->front + 1) % MAX_TASKS;
pool->count--;
pthread_cond_signal(&(pool->notify_producer));
pthread_mutex_unlock(&(pool->lock));
task.function(task.arg);
}
return NULL;
}
void task_function(void* arg) {
int task_id = (intptr_t)arg;
printf("Task %d: Executing\n", task_id);
// 在这里执行任务的具体逻辑
}
int main() {
threadpool_t pool;
threadpool_init(&pool, MAX_THREADS);
threadpool_run(&pool);
threadpool_destroy(&pool);
return 0;
}
```
以上代码实现了一个简单的固定大小的线程池,可以添加任务并由线程池中的线程执行。你可以在 `task_function` 函数中编写具体的任务逻辑,然后通过调用 `threadpool_add_task` 函数将任务添加到线程池中。
请注意,此示例代码仅为演示用途,没有处理线程池的销毁和错误处理等情况。在实际使用中,你可能需要根据自己的需求进行适当的扩展和修改。
相关推荐
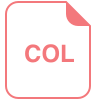
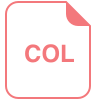
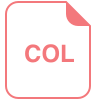
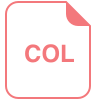
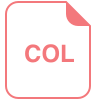









