写一个线程池用C语言
时间: 2023-02-28 20:01:29 浏览: 61
C语言中线程池的实现需要使用多线程相关的库,比如pthread库。
以下是一个简单的线程池示例:
```
#include <pthread.h>
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#define NUM_THREADS 5
void *thread_func(void *arg) {
int *id = (int*)arg;
printf("Thread %d running\n", *id);
sleep(1);
printf("Thread %d exiting\n", *id);
pthread_exit(NULL);
}
int main(int argc, char *argv[]) {
pthread_t threads[NUM_THREADS];
int thread_ids[NUM_THREADS];
for (int i = 0; i < NUM_THREADS; i++) {
thread_ids[i] = i;
int rc = pthread_create(&threads[i], NULL, thread_func, &thread_ids[i]);
if (rc) {
printf("Error creating thread %d\n", i);
exit(1);
}
}
for (int i = 0; i < NUM_THREADS; i++) {
pthread_join(threads[i], NULL);
}
return 0;
}
```
这个示例中,创建了5个线程,每个线程都调用了`thread_func`函数,并在函数中打印一些信息。
该示例仅作为简单的示例,不包括线程池的所有功能,你可以根据需要扩展它。
相关推荐
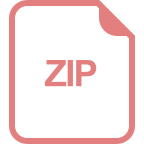
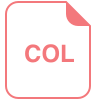
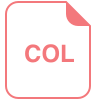
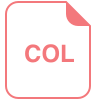
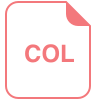
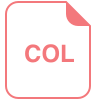









