1、 实现线性表、栈和队列(要求顺序和链式两种实现)用Java,要初学者看得懂的,简单一些简短一些的
时间: 2024-09-30 09:06:26 浏览: 27
在Java中,我们可以使用数组(数组是一种顺序结构)和链表(链式结构)分别实现线性表、栈和队列。
**1. 顺序实现(数组):**
```java
// 线性表 - 数组实现
public class ArrayLinearList {
private int[] elements;
private int size;
// 构造函数
public ArrayLinearList(int capacity) {
elements = new int[capacity];
size = 0;
}
// 添加元素到末尾
public void add(int value) {
if (size == elements.length) {
resize(size * 2);
}
elements[size++] = value;
}
// 删除末尾元素(栈)
public void pop() {
if (isEmpty()) throw new IllegalStateException();
size--;
}
// 删除首部元素(队列)
public void dequeue() {
if (isEmpty()) throw new IllegalStateException();
System.arraycopy(elements, 1, elements, 0, size - 1);
size--;
}
// 其他辅助方法...
}
```
**2. 链式实现(链表):**
```java
// 线性表 - 链表实现
public class LinkedListNode {
int data;
LinkedListNode next;
// constructor and methods...
}
public class LinkedListLinearList {
private LinkedListNode head;
// 插入节点方法
public void add(int value) {
LinkedListNode newNode = new LinkedListNode(value);
if (head == null) {
head = newNode;
} else {
LinkedListNode current = head;
while (current.next != null) {
current = current.next;
}
current.next = newNode;
}
}
// 删除头节点(栈)
public void pop() {
if (head == null) throw new IllegalStateException();
LinkedListNode temp = head;
head = head.next;
temp.next = null; // 或者可以手动释放引用
}
// 删除尾节点(队列)
public void dequeue() {
if (head == null) throw new IllegalStateException();
if (head.next == null) {
head = null;
} else {
LinkedListNode current = head;
while (current.next.next != null) {
current = current.next;
}
current.next = null;
}
}
// 其他辅助方法...
}
```
阅读全文
相关推荐
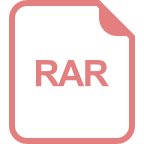
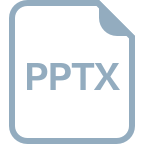
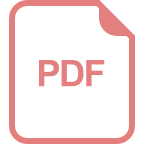
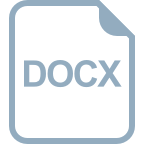
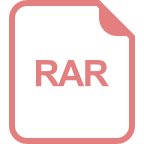
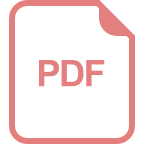
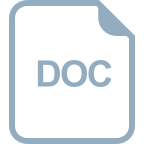
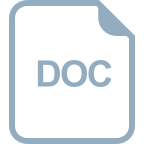
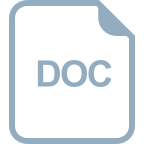
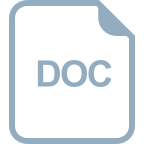
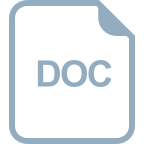
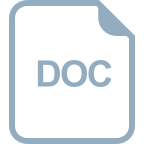
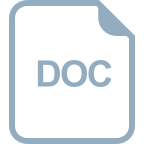
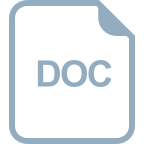
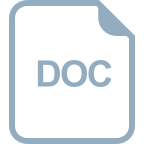
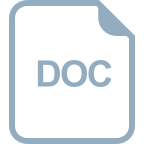
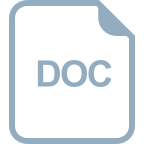
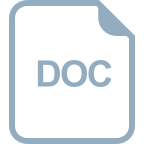
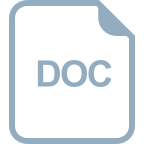