如何给卷积网络中双分支结构用pytorch实现分配可学习的权重
时间: 2024-02-20 09:59:58 浏览: 141
要实现卷积网络中的双分支结构并分配可学习的权重,可以使用PyTorch中的nn.ModuleList和nn.ParameterList。
首先,需要定义两个分支,然后将它们包含在一个nn.ModuleList中。接着,为每个分支定义一个nn.ParameterList作为可学习参数的容器。
在forward函数中,可以分别计算两个分支的输出,并使用nn.functional中的一些函数(如concatenate、add等)将它们结合起来。最后,返回最终的输出。
以下是一个示例代码:
```python
import torch
import torch.nn as nn
import torch.nn.functional as F
class DoubleBranchNet(nn.Module):
def __init__(self, in_channels, out_channels):
super(DoubleBranchNet, self).__init__()
self.branch1 = nn.Sequential(
nn.Conv2d(in_channels, 64, kernel_size=3, padding=1),
nn.BatchNorm2d(64),
nn.ReLU(inplace=True),
nn.Conv2d(64, out_channels, kernel_size=3, padding=1),
nn.BatchNorm2d(out_channels),
nn.ReLU(inplace=True)
)
self.branch2 = nn.Sequential(
nn.Conv2d(in_channels, 64, kernel_size=1),
nn.BatchNorm2d(64),
nn.ReLU(inplace=True),
nn.Conv2d(64, out_channels, kernel_size=3, padding=1),
nn.BatchNorm2d(out_channels),
nn.ReLU(inplace=True)
)
self.branch1_weights = nn.ParameterList(
[nn.Parameter(torch.randn(out_channels)) for i in range(2)]
)
self.branch2_weights = nn.ParameterList(
[nn.Parameter(torch.randn(out_channels)) for i in range(2)]
)
def forward(self, x):
out1 = self.branch1(x)
out2 = self.branch2(x)
out1 = F.conv2d(out1, self.branch1_weights[0])
out2 = F.conv2d(out2, self.branch2_weights[0])
out1 = F.conv2d(out1, self.branch1_weights[1])
out2 = F.conv2d(out2, self.branch2_weights[1])
out = torch.cat([out1, out2], dim=1)
out = F.relu(out)
return out
```
在这个示例中,我们首先定义了两个分支,每个分支都有两个卷积层和两个BatchNorm层。然后,我们为每个分支创建了一个nn.ParameterList,用于存储可学习的权重参数。
在forward函数中,我们分别计算了两个分支的输出,并使用nn.functional中的conv2d函数将它们与对应的权重参数进行卷积运算。最后,我们将它们拼接在一起,并进行一次ReLU激活,得到最终的输出。
阅读全文
相关推荐
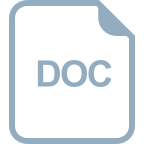
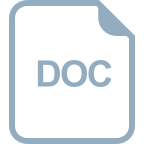
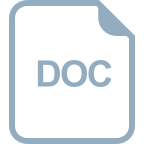
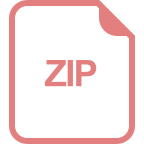
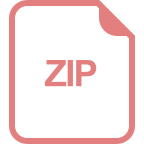
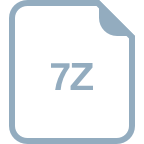
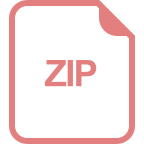
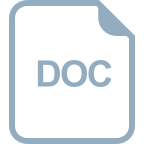
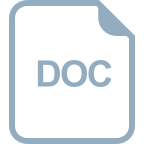
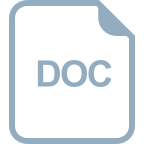
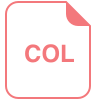
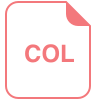
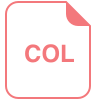
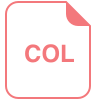
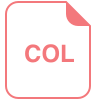
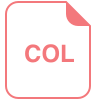
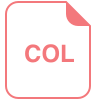
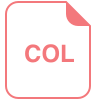