self.query_conv = nn.Conv2d(in_channels=in_dim, out_channels=in_dim // 8, kernel_size=1)
时间: 2024-05-28 19:11:39 浏览: 167
这是一个卷积层,用于将输入的特征图进行通道数的变换。其中,`in_channels`表示输入的特征图的通道数,`out_channels`表示输出的特征图的通道数,`kernel_size`表示卷积核的大小。这里采用的是1x1的卷积核,因此可以看作是一个通道数的变换。具体来说,`in_dim`表示输入特征图的通道数,`in_dim // 8`表示输出特征图的通道数为输入特征图通道数的1/8,这样做可以减少计算量,同时也可以引入更多的非线性变换。
相关问题
class Self_Attn(nn.Module): """ Self attention Layer""" def __init__(self, in_dim, activation=None): super(Self_Attn, self).__init__() # self.chanel_in = in_dim # self.activation = activation self.query_conv = nn.Conv2d(in_channels=in_dim, out_channels=in_dim // 8, kernel_size=1) self.key_conv = nn.Conv2d(in_channels=in_dim, out_channels=in_dim // 8, kernel_size=1) self.value_conv = nn.Conv2d(in_channels=in_dim, out_channels=in_dim, kernel_size=1) self.gamma = nn.Parameter(torch.zeros(1)) self.softmax = nn.Softmax(dim=-1) # def forward(self, x): """ inputs : x : input feature maps( B X C X W X H) returns : out : self attention value + input feature attention: B X N X N (N is Width*Height) """ # batch,通道数,宽,高 m_batchsize, C, width, height = x.size() # [1, 16, 32, 32] # 步骤1, 通过conv 得出q,k q = self.query_conv(x).view(m_batchsize, -1, width * height).permute(0, 2, 1) # B X CX(N) torch.Size([1, 1024, 2]) k = self.key_conv(x).view(m_batchsize, -1, width * height) # B X C x (*W*H) torch.Size([1, 2, 1024]) # 步骤1, 计算得出v v = self.value_conv(x).view(m_batchsize, -1, width * height) # B X C X N torch.Size([1, 16, 1024]) # 步骤2, 矩阵的乘法 ,q,k进行相乘,得出特征图 # [batch_size,1024,2]*[batch_size,2,1024] energy = torch.bmm(q, k) # transpose check [1, 1024, 1024] # 特征图attention map,通过softmax attention = self.softmax(energy) # BX (N) X (N) torch.Size([1, 1024, 1024]) # 步骤3,v * 特征图= 注意力 # [1,16,1024] * [1,1024,1024]= torch.Size([1, 16, 1024]) out = torch.bmm(v, attention.permute(0, 2, 1)) # torch.Size([1, 16, 1024]) # 重新resize out = out.view(m_batchsize, C, width, height) # torch.Size([1, 16, 32, 32]) # 加上残差 out = self.gamma * out + x return out
这段代码实现了一个Self Attention Layer,其中包含三个卷积层,分别是query_conv、key_conv和value_conv,用于计算注意力的query、key和value。在前向传播中,首先通过query_conv和key_conv计算出query和key,然后计算出注意力矩阵,最后通过value_conv将注意力矩阵与输入特征图相乘得到注意力值,并加上残差。它可以用于图像、文本等任务中,用于提取输入中的重要信息。
self-attention gan 代码_GAN+异常检测
以下是 Self-Attention GAN 代码和 GAN+异常检测的代码示例:
Self-Attention GAN 代码:
```
import torch.nn as nn
import torch
class SelfAttention(nn.Module):
def __init__(self, in_channels):
super(SelfAttention, self).__init__()
self.query_conv = nn.Conv2d(in_channels=in_channels, out_channels=in_channels // 8, kernel_size=1)
self.key_conv = nn.Conv2d(in_channels=in_channels, out_channels=in_channels // 8, kernel_size=1)
self.value_conv = nn.Conv2d(in_channels=in_channels, out_channels=in_channels, kernel_size=1)
self.gamma = nn.Parameter(torch.zeros(1))
def forward(self, x):
m_batchsize, C, width, height = x.size()
proj_query = self.query_conv(x).view(m_batchsize, -1, width * height).permute(0, 2, 1)
proj_key = self.key_conv(x).view(m_batchsize, -1, width * height)
energy = torch.bmm(proj_query, proj_key)
attention = torch.softmax(energy, dim=-1)
proj_value = self.value_conv(x).view(m_batchsize, -1, width * height)
out = torch.bmm(proj_value, attention.permute(0, 2, 1))
out = out.view(m_batchsize, C, width, height)
out = self.gamma * out + x
return out
```
GAN+异常检测代码:
```
import torch.nn as nn
import torch
import numpy as np
class Generator(nn.Module):
def __init__(self, latent_dim, img_shape):
super(Generator, self).__init__()
self.img_shape = img_shape
def block(in_feat, out_feat, normalize=True):
layers = [nn.Linear(in_feat, out_feat)]
if normalize:
layers.append(nn.BatchNorm1d(out_feat, 0.8))
layers.append(nn.LeakyReLU(0.2, inplace=True))
return layers
self.model = nn.Sequential(
*block(latent_dim, 128, normalize=False),
*block(128, 256),
*block(256, 512),
*block(512, 1024),
nn.Linear(1024, int(np.prod(img_shape))),
nn.Tanh()
)
def forward(self, z):
img = self.model(z)
img = img.view(img.size(0), *self.img_shape)
return img
class Discriminator(nn.Module):
def __init__(self, img_shape):
super(Discriminator, self).__init__()
self.img_shape = img_shape
self.model = nn.Sequential(
nn.Linear(int(np.prod(img_shape)), 512),
nn.LeakyReLU(0.2, inplace=True),
nn.Linear(512, 256),
nn.LeakyReLU(0.2, inplace=True),
nn.Linear(256, 1),
nn.Sigmoid(),
)
def forward(self, img):
img_flat = img.view(img.size(0), -1)
validity = self.model(img_flat)
return validity
class GAN_Anomaly_Detector(nn.Module):
def __init__(self, latent_dim, img_shape):
super(GAN_Anomaly_Detector, self).__init__()
self.generator = Generator(latent_dim, img_shape)
self.discriminator = Discriminator(img_shape)
def forward(self, x):
z = torch.randn(x.shape[0], LATENT_DIM, device=device)
gen_imgs = self.generator(z)
validity_real = self.discriminator(x)
validity_fake = self.discriminator(gen_imgs)
return torch.mean(torch.abs(x - gen_imgs)) + valid_loss(validity_real, validity_fake)
def valid_loss(validity_real, validity_fake):
real_loss = nn.functional.binary_cross_entropy(validity_real, torch.ones_like(validity_real))
fake_loss = nn.functional.binary_cross_entropy(validity_fake, torch.zeros_like(validity_fake))
return (real_loss + fake_loss) / 2
```
这里的 GAN+异常检测是通过计算生成图像与输入图像之间的差异以及判别器的输出来进行异常检测。如果生成的图像与输入图像越接近,则相似度越高,否则就是异常。
阅读全文
相关推荐
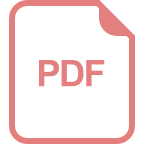
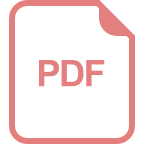






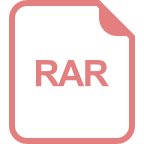
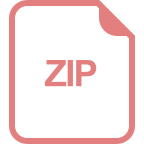
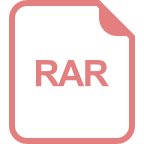
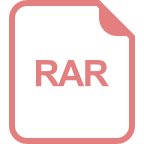
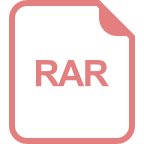