self attention pytorch 在图像中运用的代码
时间: 2023-07-27 13:06:59 浏览: 51
Self-Attention 机制最初是在自然语言处理领域中使用的,但是它也可以被应用在计算机视觉中,例如图像分类、图像分割、目标检测等任务中。
在 PyTorch 中,可以使用 `torch.nn.MultiheadAttention` 模块来实现 Self-Attention 机制。下面是一个使用 Self-Attention 机制进行图像分类的示例代码:
```python
import torch
import torch.nn as nn
import torch.nn.functional as F
class SelfAttention(nn.Module):
def __init__(self, in_channels, out_channels):
super(SelfAttention, self).__init__()
self.out_channels = out_channels
self.query_conv = nn.Conv2d(in_channels, out_channels, kernel_size=1)
self.key_conv = nn.Conv2d(in_channels, out_channels, kernel_size=1)
self.value_conv = nn.Conv2d(in_channels, out_channels, kernel_size=1)
self.gamma = nn.Parameter(torch.zeros(1))
def forward(self, x):
batch_size, channels, height, width = x.size()
query = self.query_conv(x).view(batch_size, self.out_channels, -1)
key = self.key_conv(x).view(batch_size, self.out_channels, -1)
value = self.value_conv(x).view(batch_size, self.out_channels, -1)
attention_weights = F.softmax(torch.bmm(query.transpose(1, 2), key), dim=2)
attention_output = torch.bmm(value, attention_weights.transpose(1, 2))
attention_output = attention_output.view(batch_size, self.out_channels, height, width)
out = self.gamma * attention_output + x
return out
class Net(nn.Module):
def __init__(self):
super(Net, self).__init__()
self.conv1 = nn.Conv2d(3, 64, kernel_size=3, padding=1)
self.sa1 = SelfAttention(64, 64)
self.conv2 = nn.Conv2d(64, 128, kernel_size=3, padding=1)
self.sa2 = SelfAttention(128, 128)
self.conv3 = nn.Conv2d(128, 256, kernel_size=3, padding=1)
self.sa3 = SelfAttention(256, 256)
self.pool = nn.MaxPool2d(2, 2)
self.fc1 = nn.Linear(256 * 4 * 4, 1024)
self.fc2 = nn.Linear(1024, 10)
def forward(self, x):
x = F.relu(self.conv1(x))
x = self.sa1(x)
x = self.pool(x)
x = F.relu(self.conv2(x))
x = self.sa2(x)
x = self.pool(x)
x = F.relu(self.conv3(x))
x = self.sa3(x)
x = self.pool(x)
x = x.view(-1, 256 * 4 * 4)
x = F.relu(self.fc1(x))
x = self.fc2(x)
return x
```
这个示例代码定义了一个包含 Self-Attention 机制的卷积神经网络(CNN)用于图像分类。`SelfAttention` 类表示 Self-Attention 模块,它接受输入张量 `x`,并返回经过 Self-Attention 机制处理后的输出张量。`Net` 类表示整个 CNN,它包含了三个卷积层和三个 Self-Attention 模块。在每个卷积层之后都使用了一个池化层,并且在最后的全连接层之前使用了一个展平层。在 `forward` 方法中,首先对输入进行卷积操作,然后使用 Self-Attention 模块进行特征提取,接着进行池化操作,最后进行全连接操作得到分类结果。
相关推荐
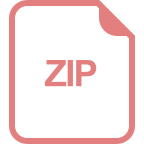
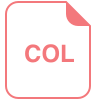
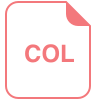
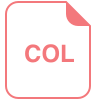

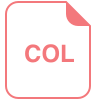








