写一个基于JPEG2000的彩色图像压缩,在jupyter notebooks上运行,能设置不同的压缩比
时间: 2024-02-17 22:04:55 浏览: 68
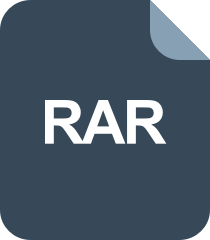
基于JPEG的图像压缩系统
好的,我了解了您的要求。对于基于JPEG2000的彩色图像压缩,我们可以使用Python的开源库pywavelets来实现。
首先,我们需要安装pywavelets库。在Jupyter Notebook中运行以下命令:
```python
!pip install pywavelets
```
接下来,我们可以编写以下代码来实现基于JPEG2000的彩色图像压缩:
```python
import pywt
import numpy as np
from PIL import Image
def compress_image(image_path, compression_ratio):
# 打开图像文件
image = Image.open(image_path)
# 将图像转换为numpy数组
image_array = np.array(image)
# 分离RGB通道
r = image_array[:,:,0]
g = image_array[:,:,1]
b = image_array[:,:,2]
# 对每个通道进行小波变换
coeffs_r = pywt.dwt2(r, 'haar')
coeffs_g = pywt.dwt2(g, 'haar')
coeffs_b = pywt.dwt2(b, 'haar')
# 将小波系数按照压缩比进行截断
threshold_r = np.max(coeffs_r[0]) * compression_ratio
threshold_g = np.max(coeffs_g[0]) * compression_ratio
threshold_b = np.max(coeffs_b[0]) * compression_ratio
coeffs_r = pywt.threshold(coeffs_r, threshold_r, mode='hard')
coeffs_g = pywt.threshold(coeffs_g, threshold_g, mode='hard')
coeffs_b = pywt.threshold(coeffs_b, threshold_b, mode='hard')
# 对每个通道进行小波反变换
compressed_r = pywt.idwt2(coeffs_r, 'haar')
compressed_g = pywt.idwt2(coeffs_g, 'haar')
compressed_b = pywt.idwt2(coeffs_b, 'haar')
# 将RGB通道合并
compressed_image_array = np.zeros_like(image_array)
compressed_image_array[:,:,0] = compressed_r
compressed_image_array[:,:,1] = compressed_g
compressed_image_array[:,:,2] = compressed_b
# 将numpy数组转换为图像
compressed_image = Image.fromarray(np.uint8(compressed_image_array))
return compressed_image
```
上述代码中,我们首先打开图像文件,并将其转换为numpy数组。然后,我们对每个通道进行小波变换,并根据压缩比进行截断。最后,我们对每个通道进行小波反变换,并将RGB通道合并。最终,我们将压缩后的图像保存为PIL Image对象并返回。
我们可以使用以下代码来测试上述函数:
```python
# 压缩比为0.5
compressed_image = compress_image('test.jpg', 0.5)
compressed_image.save('compressed.jpg')
```
在上述代码中,我们将压缩比设置为0.5,并将压缩后的图像保存为名为“compressed.jpg”的文件。
希望这可以解决您的问题!
阅读全文
相关推荐















