如何从C++代码vector<CityData> readDataFromExcel() ,读取地址"C:\Users\86139\Desktop\主成分作业 数据.csv"
时间: 2023-09-10 16:12:35 浏览: 50
你可以使用第三方库来读取Excel文件,比如LibXL,但这个库是商业授权的。如果你不想使用商业授权的库,那么你可以使用开源库,比如libcsv或者TinyCsvParser。
下面是一个使用TinyCsvParser库读取CSV文件的示例代码:
```c++
#include <iostream>
#include <string>
#include <vector>
#include "tinycsvparser.hpp"
struct CityData {
std::string name;
int population;
double latitude;
double longitude;
};
int main() {
// Define the CSV parser settings
const char* filename = "C:\\Users\\86139\\Desktop\\主成分作业 数据.csv";
const char delimiter = ',';
const int numFields = 4;
// Define the data structure to store the parsed CSV data
typedef CityData CsvData;
std::vector<CsvData> csvData;
// Define the fields in the CSV file
struct CsvFields {
std::string name;
int population;
double latitude;
double longitude;
} fields;
// Define the CSV parser
CsvParserSettings parserSettings(numFields);
parserSettings.delimiter = delimiter;
CsvMapping<CsvFields> mapping(
fields.name, "name",
fields.population, "population",
fields.latitude, "latitude",
fields.longitude, "longitude");
parserSettings.mapping = mapping;
// Create the CSV parser
CsvParser<CsvFields> parser(parserSettings);
// Parse the CSV file and store the data in the vector
parser.read_csv(filename, [&](const CsvFields& f) {
csvData.push_back(CityData{ f.name, f.population, f.latitude, f.longitude });
});
// Print the parsed data
for (const auto& data : csvData) {
std::cout << data.name << ", " << data.population << ", "
<< data.latitude << ", " << data.longitude << std::endl;
}
return 0;
}
```
这个示例代码假设CSV文件的第一行包含了4个字段:name、population、latitude和longitude。如果你的CSV文件包含不同的字段,需要修改CsvFields结构体和CsvMapping模板参数。
另外需要注意的是,TinyCsvParser库使用C++11特性,需要在编译时加上-std=c++11选项。
相关推荐
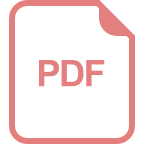














