写一份串口通信,缓冲区为4096大小的c语言代码
时间: 2023-05-27 15:04:19 浏览: 70
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <fcntl.h>
#include <termios.h>
#define BUF_SIZE 4096 // 缓冲区大小
int main(int argc, char *argv[]) {
if (argc != 2) {
fprintf(stderr, "Usage: %s <serial port device>\n", argv[0]);
exit(1);
}
char *serial_port = argv[1];
int serial_fd = open(serial_port, O_RDWR | O_NOCTTY | O_NDELAY);
if (serial_fd == -1) {
fprintf(stderr, "Error: Cannot open serial port %s\n", serial_port);
exit(1);
}
struct termios options;
tcgetattr(serial_fd, &options); // 获取当前串口配置
options.c_cflag = B9600 | CS8 | CLOCAL | CREAD;
options.c_iflag = IGNPAR | ICRNL;
options.c_oflag = 0;
options.c_lflag = ICANON;
tcflush(serial_fd, TCIFLUSH);
tcsetattr(serial_fd, TCSANOW, &options); // 配置串口
char buf[BUF_SIZE];
int buf_len = 0;
while (1) {
// 读取串口数据
int n = read(serial_fd, buf + buf_len, BUF_SIZE - buf_len);
if (n == -1) {
fprintf(stderr, "Error: Cannot read from serial port\n");
break;
}
buf_len += n;
// 处理缓冲区数据
char *p = buf;
while (buf_len >= 6) {
if (strncmp(p, "START", 5) == 0) { // 如果接收到"START",回复"ACK"
write(serial_fd, "ACK", 3);
p += 5;
buf_len -= 5;
} else if (strncmp(p, "STOP", 4) == 0) { // 如果接收到"STOP",退出程序
break;
} else { // 否则跳过一字节
p++;
buf_len--;
}
}
// 重置缓冲区
if (buf_len > 0 && p != buf) {
memmove(buf, p, buf_len);
} else {
buf_len = 0;
}
}
close(serial_fd);
return 0;
}
```
相关推荐
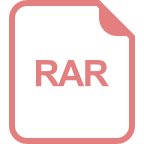
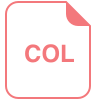
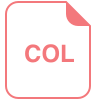
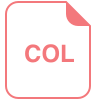
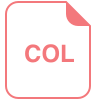










