如何用C++编程实现21点游戏?
时间: 2024-10-29 17:19:28 浏览: 13
21点(也称Blackjack),是一种流行的纸牌游戏,通常用于演示计算机算法,尤其是策略和概率。在C++中实现21点的基本步骤如下:
1. 定义数据结构:创建一个Deck类表示整副牌,包含各种花色(红桃、黑桃、梅花、方块)和数值(A到K各有对应的点数)。
```cpp
class Card {
public:
enum Suit { SPADES, DIAMONDS, CLUBS, HEARTS };
int value;
};
```
2. 创建Deck类:负责洗牌和发牌功能,以及计算剩余牌堆的大小。
```cpp
class Deck {
private:
std::vector<Card> cards;
public:
void shuffle();
Card dealCard();
size_t getRemainingCards() const;
};
```
3. 实现玩家和庄家:Player和Dealer类,每个都有手牌(hands)属性,并能接收新牌、显示当前总点数等。
4. 游戏规则逻辑:在Game类中,制定游戏循环,让玩家和庄家轮流取牌,直到达到爆牌线(超过21点或玩家选择停止)。
```cpp
class Game {
private:
Player player;
Dealer dealer;
public:
void playRound();
bool isGameOver();
// 其他辅助函数...
};
```
5. 主函数:初始化游戏环境,开始游戏循环,处理用户输入,判断胜负并更新分数。
```cpp
int main() {
Deck deck;
deck.shuffle();
while (!Game().isGameOver()) {
Game().playRound();
// 更新得分...
}
return 0;
}
```
阅读全文
相关推荐
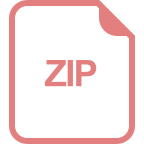
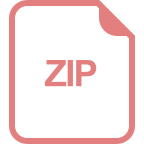
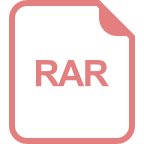
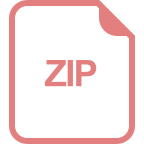
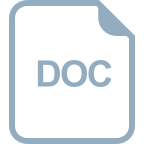
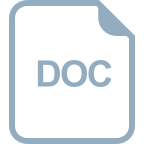
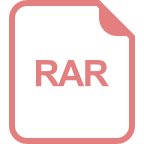
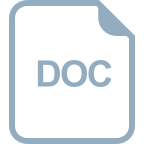
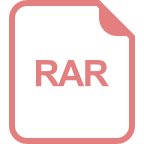
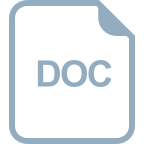
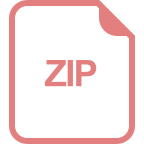
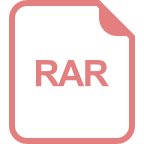
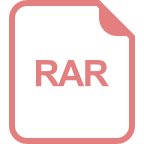
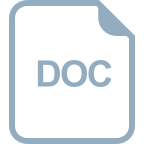
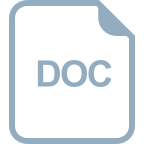
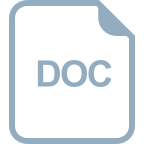
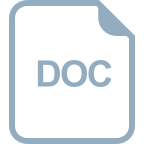
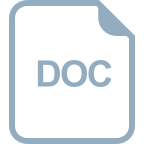