编写一个算法,在头结点为h的单链表中,把值为b的结点插入到值为a的结点之前,若不存在a,则把结点s插入到表尾。
时间: 2024-09-29 07:05:50 浏览: 27
为了在给定的单链表中插入节点,我们可以按照以下步骤编写一个算法:
1. 定义一个辅助函数 `findNode(head, value)`,用于查找目标值 `value` 的节点。如果找到该节点,返回它;如果没有找到,则返回 `nullptr` 或 `NULL` 表示未找到。
```cpp
Node* findNode(Node* head, int a) {
Node* current = head;
while (current != nullptr && current->data != a) {
current = current->next;
}
return current;
}
```
2. 创建一个新的节点 `newNode` 并设置其值为 `b` 和指针为 `nullptr`。
3. 分别处理三种情况:
- 如果要将 `b` 插入到 `a` 之前,首先找到 `a` 节点,然后将 `newNode` 插入在其前。
- 如果 `a` 不存在,说明要在表尾插入 `s` 或 `b`,直接将 `newNode` 设置为新链表的头。
- 如果 `a` 存在并且要插入 `b`,则将 `a` 的前一个节点的 `next` 指向 `newNode`,然后 `newNode` 的 `next` 指向 `a`。
以下是完整的 C++ 代码实现:
```cpp
void insertBeforeAfter(Node** h, Node* s, int a, int b) {
// Step 1: Create the new node
Node* newNode = new Node(b);
// Step 2: Find the target nodes
Node* aNode = findNode(*h, a);
Node* lastNode = nullptr;
// Step 3: Insert based on conditions
if (aNode != nullptr) {
newNode->next = aNode; // If a exists and b should be inserted before it
aNode->prev = newNode;
if (lastNode == nullptr) { // If this is also the first node
*h = newNode;
} else {
lastNode->next = newNode;
}
} else {
if (*h == nullptr || s == nullptr) {
// If no existing node or s is null, insert at the beginning
*h = newNode;
newNode->next = s;
} else {
newNode->next = s; // If s exists, insert at the end
if (s->prev != nullptr) {
s->prev->next = newNode;
} else {
*h = newNode; // s is the first node
}
s->prev = newNode;
}
}
delete s; // Delete the old temporary 's' node if provided
}
```
阅读全文
相关推荐
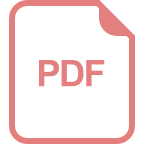
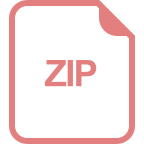
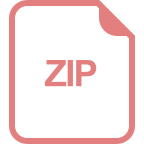















