一元多项式求导c++
时间: 2024-01-04 09:19:17 浏览: 129
以下是一元多项式求导的C++代码示例:
```cpp
#include <iostream>
using namespace std;
int main() {
int a, b, flag = 0; // 设flag
while (cin >> a >> b) {
if (b != 0) {
if (flag == 1) // 已有输出
cout << " ";
cout << a * b << " " << b - 1;
flag = 1;
}
}
if (flag == 0) // 导数为零多项式
cout << "0 0";
return 0;
}
```
该代码通过输入一元多项式的系数和指数,计算并输出其导数的系数和指数。其中,如果导数为零多项式,则输出"0 0";如果导数不为零多项式,则输出每一项的系数和指数。
相关问题
1010 一元多项式求导map
### 关于一元多项式求导的实现
在一元多项式的表示和操作中,`map` 是一种非常合适的数据结构来存储系数及其对应的指数。因为 `map` 可以自动按照键(即指数)进行排序,并且支持高效的插入、删除和查找操作。
#### 使用 C++ 的 std::map 来实现一元多项式求导
下面是一个完整的例子,展示如何利用 C++ 中的标准库容器 `std::map<int, int>` 来表示一个一元多项式,并对其进行求导:
```cpp
#include <iostream>
#include <map>
using namespace std;
// 定义多项式类
class Polynomial {
private:
map<int, double> terms; // 存储项 {exponent -> coefficient}
public:
void addTerm(int exponent, double coefficient);
friend ostream& operator<<(ostream&, const Polynomial&);
Polynomial derivative() const;
};
void Polynomial::addTerm(int exponent, double coefficient) {
if (coefficient != 0)
terms[exponent] += coefficient;
}
ostream& operator<<(ostream &out, const Polynomial &p) {
bool first = true;
for (auto it = p.terms.rbegin(); it != p.terms.rend(); ++it) {
if (!first) out << " + ";
if (it->second != 1 || it->first == 0) out << it->second;
if (it->first > 0) out << "x";
if (it->first >= 2) out << "^" << it->first;
first = false;
}
return out;
}
Polynomial Polynomial::derivative() const {
Polynomial result;
for (const auto &[exp, coeff] : terms) {
if (exp > 0) {
result.addTerm(exp - 1, exp * coeff);
}
}
return result;
}
int main() {
Polynomial poly;
// 添加一些项到多项式中
poly.addTerm(3, 4); // 4x^3
poly.addTerm(2, -5); // -5x^2
poly.addTerm(1, 7); // 7x
poly.addTerm(0, 9); // 9
cout << "原始多项式:" << poly << endl;
cout << "求导后的多项式:" << poly.derivative() << endl;
return 0;
}
```
这段程序定义了一个名为 `Polynomial` 的类,该类内部使用 `std::map<int, double>` 来保存每一项的指数作为 key 和其相应的系数作为 value 。通过成员函数 `addTerm()` 向多项式添加新项;重载了流插入运算符以便能够方便地打印多项式表达式;最后实现了 `derivative()` 方法用于计算给定多项式的导数[^2]。
一元多项式的求导c++代码
下面是一元多项式的求导的 C++ 代码:
```c++
#include <iostream>
#include <vector>
using namespace std;
// 定义一个结构体来表示多项式的每一项
struct Term {
int coef; // 系数
int exp; // 指数
};
// 多项式求导函数
vector<Term> derivative(vector<Term>& poly) {
vector<Term> result; // 存储求导后的多项式
// 遍历多项式的每一项,求导并加入到结果中
for (auto term : poly) {
if (term.exp > 0) { // 指数大于0才有导数
Term dTerm = {term.coef * term.exp, term.exp - 1};
result.push_back(dTerm);
}
}
return result;
}
// 输出多项式
void printPoly(vector<Term>& poly) {
for (int i = 0; i < poly.size(); i++) {
if (i > 0 && poly[i].coef > 0) cout << "+"; // 输出符号
cout << poly[i].coef;
if (poly[i].exp > 0) cout << "x^" << poly[i].exp; // 输出指数
}
cout << endl;
}
int main() {
// 定义一个多项式,这里用一个 vector 来存储多项式的每一项
vector<Term> poly = {{2, 3}, {4, 2}, {3, 1}, {5, 0}};
cout << "原多项式:";
printPoly(poly);
// 求导
vector<Term> dPoly = derivative(poly);
cout << "求导后的多项式:";
printPoly(dPoly);
return 0;
}
```
这个代码定义了一个 `Term` 结构体来表示多项式的每一项,然后用一个 vector 来存储整个多项式,`derivative` 函数接受一个多项式的 vector,返回求导后的多项式的 vector。最后通过 `printPoly` 函数来输出多项式的表达式。在 `main` 函数中,我们先定义一个多项式,然后调用 `derivative` 函数来求导,并分别输出原多项式和求导后的多项式。
阅读全文
相关推荐


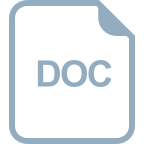








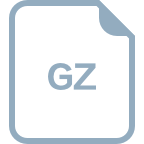


