python dataframe按照分隔符一列分为多列
时间: 2024-05-28 16:07:21 浏览: 24
在Python中,可以使用pandas库来处理数据,其中DataFrame是一种常用的数据结构。如果想将DataFrame中的一列按照某个分隔符拆分成多列,可以使用pandas中的str.split()方法。
具体步骤如下:
1. 读取数据并创建DataFrame对象。
2. 使用str.split()方法对需要拆分的列进行处理,将其转换为多列,可以指定分隔符和拆分后的列数。
3. 将拆分后的列添加到原DataFrame中,并删除原列。
示例代码如下:
```python
import pandas as pd
# 创建DataFrame对象
df = pd.DataFrame({'col1': ['a|b|c', 'd|e|f']})
# 使用str.split()方法将col1列按照"|"分隔为三列
df[['col2', 'col3', 'col4']] = df['col1'].str.split('|', expand=True)
# 删除原列
df.drop('col1', axis=1, inplace=True)
print(df)
```
输出结果如下:
```
col2 col3 col4
0 a b c
1 d e f
```
相关问题
python dataframe 多列拼成一列
在Python的pandas库中,可以使用concat方法将多个列拼接成一列。以下是示例代码:
```python
import pandas as pd
# 创建一个包含多个列的DataFrame
df = pd.DataFrame({'col1': [1, 2, 3], 'col2': [4, 5, 6], 'col3': [7, 8, 9]})
# 将多个列拼接成一列
df['new_col'] = df['col1'].astype(str) + '-' + df['col2'].astype(str) + '-' + df['col3'].astype(str)
# 打印结果
print(df)
```
在上面的示例中,我们使用`concat`方法将`col1`、`col2`和`col3`三列拼接成了一列`new_col`。在拼接时,我们使用了字符串连接操作符`+`将三列的值连接起来,并在值之间添加了分隔符`-`。需要注意的是,由于拼接后的新列中的值是字符串类型,因此我们需要使用`astype(str)`方法将原始列的数值转换成字符串类型。
dataframe一列拆分成多列
要将dataframe的一列拆分成多列,可以使用split函数结合expand参数来实现。首先,使用split函数将列的值按照指定的分隔符拆分成多个子字符串,并将结果转换成一个新的dataframe。然后,使用concat函数将新的dataframe与原来的dataframe进行合并。最后,使用rename函数给新的列名进行重命名。下面是一种完整的操作过程:<em>1</em><em>2</em><em>3</em>
#### 引用[.reference_title]
- *1* *3* [Python Pandas:DataFrame 一列切分成多列、分隔符切分选字段](https://blog.csdn.net/baidu_40468340/article/details/128263235)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v92^chatsearchT3_1"}} ] [.reference_item]
- *2* [Dataframe一列分割成多列](https://blog.csdn.net/weixin_46599926/article/details/122985897)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v92^chatsearchT3_1"}} ] [.reference_item]
[ .reference_list ]
相关推荐
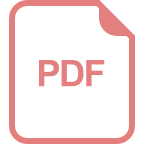
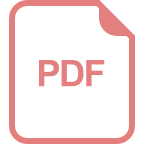
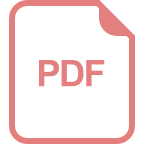












