写一篇完整的单链表代码
时间: 2024-10-22 07:21:10 浏览: 13
当然,这里是一个完整的单链表实现,包括了节点的定义、链表的构造、插入节点、删除节点以及遍历链表的基本功能。我们将使用C++语言编写:
```cpp
#include <iostream>
// 定义链表节点
struct ListNode {
int val; // 数据域
ListNode* next; // 指向下一个节点的指针
ListNode(int x) : val(x), next(NULL) {} // 构造函数
};
// 定义链表类
class LinkedList {
private:
ListNode* head; // 链表头指针
public:
// 构造函数,初始化链表为空
LinkedList() : head(nullptr) {}
// 向链表头部插入节点
void insertAtFront(int value) {
ListNode* newNode = new ListNode(value);
newNode->next = head;
head = newNode;
}
// 删除给定值的第一个匹配项
void remove(int value) {
if (!head) return;
if (head->val == value) {
ListNode* temp = head;
head = head->next;
delete temp;
return;
}
ListNode* current = head;
while (current->next && current->next->val != value) {
current = current->next;
}
if (current->next) {
ListNode* toDelete = current->next;
current->next = current->next->next;
delete toDelete;
}
}
// 打印链表
void display() {
ListNode* temp = head;
while (temp) {
std::cout << temp->val << " ";
temp = temp->next;
}
std::cout << "\n";
}
// 查找是否存在特定值
bool contains(int value) {
ListNode* current = head;
while (current) {
if (current->val == value)
return true;
current = current->next;
}
return false;
}
// 添加一个新节点到链表尾部
void append(int value) {
if (!head) {
head = new ListNode(value);
return;
}
ListNode* current = head;
while (current->next) {
current = current->next;
}
current->next = new ListNode(value);
}
};
int main() {
LinkedList list;
list.insertAtFront(5);
list.append(3);
list.insertAtFront(7);
list.display(); // 输出: 7 5 3
list.remove(5);
list.display(); // 输出: 7 3
std::cout << "Does the list contain 3? " << (list.contains(3) ? "Yes" : "No") << std::endl; // 输出: Yes
return 0;
}
```
以上代码实现了单链表的基本操作,你可以根据需要扩展其他功能。
阅读全文
相关推荐
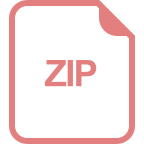
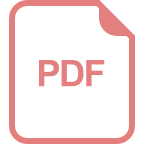
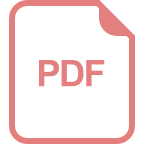

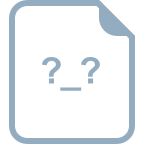
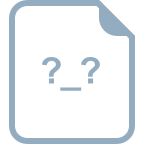
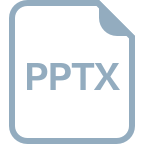
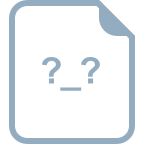
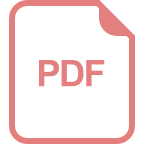
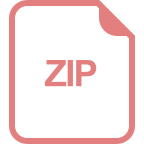
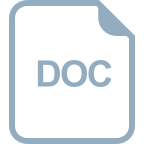






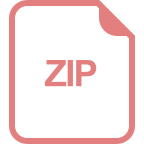