请编程实现下述操作: 1. 请以“后插法”创建一个数据元素为整数的单链表。 2. 请编写一个print函数输出单链表中的所有整数。 3. 请编写一个Max函数获取单链表中包含的最大整数值。用c++编写,并注释
时间: 2024-10-13 20:17:08 浏览: 61
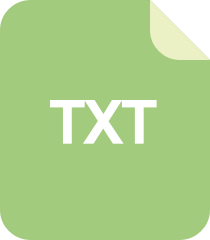
数据结构第一次上机 单链表 前插 后插多个元素 查找 删除(考虑多个元素)
在C++中,我们可以使用结构体表示链表节点,以及三个函数分别用于插入节点、打印链表和查找最大值。以下是使用后插法创建链表、打印节点以及找到最大值的代码:
```cpp
#include <iostream>
// 定义链表节点结构
struct ListNode {
int val; // 节点值
ListNode* next; // 指向下一个节点的指针
ListNode(int x) : val(x), next(nullptr) {} // 构造函数,初始化值和next为nullptr
};
// 后插法添加节点到链表
ListNode* insertNode(ListNode*& head, int val) {
// 如果链表为空,直接插入新节点作为头节点
if (head == nullptr) {
head = new ListNode(val);
return head;
}
// 否则,将新节点插入到最后
ListNode* newNode = new ListNode(val);
ListNode* current = head;
while (current->next != nullptr) {
current = current->next;
}
current->next = newNode;
return head;
}
// 打印链表中的所有整数
void printList(ListNode* head) {
if (head == nullptr) {
std::cout << "链表为空\n";
return;
}
ListNode* temp = head;
while (temp != nullptr) {
std::cout << temp->val << " ";
temp = temp->next;
}
std::cout << "\n";
}
// 获取链表中的最大值
int findMax(ListNode* head) {
if (head == nullptr) {
throw std::runtime_error("链表为空");
}
int maxVal = head->val;
ListNode* current = head->next;
while (current != nullptr) {
if (current->val > maxVal) {
maxVal = current->val;
}
current = current->next;
}
return maxVal;
}
int main() {
// 创建示例链表
ListNode* head = nullptr;
insertNode(head, 5);
insertNode(head, 3);
insertNode(head, 9);
insertNode(head, 1);
std::cout << "链表内容:\n";
printList(head);
std::cout << "链表中的最大值: " << findMax(head) << "\n";
return 0;
}
```
阅读全文
相关推荐
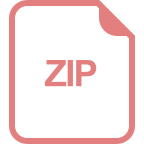





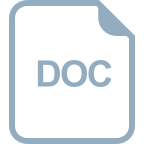
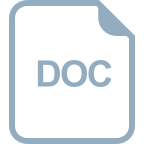









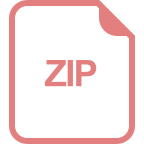