编程实现二叉树的创建、二叉树的先序、中序、后序遍历方式(递归)、二叉树的销毁。 要求: (1)从终端输入二叉树元素序列,建立对应的二叉树(先序方式); (2)采用递归的方式先序遍历、中序遍历和后序遍历二叉树并输出遍历序列; (3)采用递归方式销毁二叉树(后序方式)。
时间: 2024-10-28 18:07:12 浏览: 55
在编程中,我们可以使用递归来构建和操作二叉树。首先,我们创建二叉树的基本步骤如下:
1. **创建二叉树**:
- 先序遍历(根-左-右)适合从用户输入构建二叉树,因为可以依次插入节点:对于每个输入值,如果它不是空值,就创建一个新的节点并将当前值设为其值,然后将其作为左子节点或右子节点添加到已有的节点。
2. **遍历二叉树**:
- **先序遍历(递归)**:
```python
def preorder_traversal(root):
if root is not None:
print(root.val) # 输出节点值
preorder_traversal(root.left)
preorder_traversal(root.right)
```
- **中序遍历(递归)**:
```python
def inorder_traversal(root):
if root is not None:
inorder_traversal(root.left)
print(root.val) # 输出节点值
inorder_traversal(root.right)
```
- **后序遍历(递归)**:
```python
def postorder_traversal(root):
if root is not None:
postorder_traversal(root.left)
postorder_traversal(root.right)
print(root.val) # 输出节点值
```
3. **销毁二叉树(后序遍历递归)**:
因为二叉树的销毁通常是通过释放节点内存实现的,而在大多数语言中,这是自动的,比如在Python里,当你不再引用某个节点时,其占用的空间会被垃圾回收机制回收。不过,如果你想手动模拟这个过程,可以编写一个后序遍历的过程,最后设置根节点为空,表示树已经销毁:
```python
def destroy_tree(root):
if root is not None:
destroy_tree(root.left)
destroy_tree(root.right)
# 这里不做实际的销毁操作,仅示意图:
root = None
```
阅读全文
相关推荐



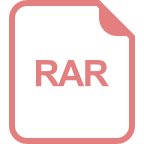










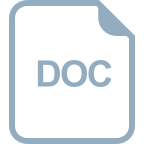
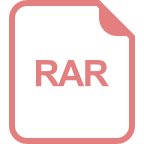
