aes128加密c++
时间: 2023-07-24 21:02:26 浏览: 146
### 回答1:
AES(Advanced Encryption Standard)是一种常见的对称加密算法,其中AES-128是使用128比特密钥进行加密的一种具体实现。
在C语言中,我们可以使用一些密码学库或者算法实现来进行AES-128加密。
一种常见的方法是使用OpenSSL库,它提供了丰富的密码学函数和工具。首先,我们需要引入`<openssl/aes.h>`头文件。然后,我们需要定义一个128比特的密钥,并将其转换为AES_KEY类型。
之后,我们可以使用AES_encrypt函数将明文数据进行加密。该函数需要传入明文数据、密钥和用于存储密文数据的缓冲区。加密后的密文数据将被写入缓冲区。
下面是一个使用OpenSSL库进行AES-128加密的简单示例:
```
#include <stdio.h>
#include <openssl/aes.h>
int main() {
unsigned char *plaintext = (unsigned char *)"This is a plaintext";
unsigned char key[16] = "AES_key123";
unsigned char ciphertext[16];
AES_KEY aes_key;
AES_set_encrypt_key(key, 128, &aes_key);
AES_encrypt(plaintext, ciphertext, &aes_key);
printf("Ciphertext: ");
for (int i = 0; i < sizeof(ciphertext); i++) {
printf("%02x", ciphertext[i]);
}
printf("\n");
return 0;
}
```
以上示例代码将明文数据"This is a plaintext"使用AES-128加密,并打印出密文数据的十六进制形式。
当然,这只是一个简单的示例,实际应用中可能需要考虑更多的问题,例如填充方式、数据块模式、密钥管理等。因此,在实际使用中,建议使用成熟的密码学库或者调用专门的加密API来确保安全性和正确性。
### 回答2:
AES128是一种对称加密算法,它可以用于保护计算机系统和数据的安全性。在C语言中,可以使用一些函数库或者API来实现AES128加密。以下是一个使用C语言进行AES128加密的简单示例:
首先,需要引入相关的加密库。例如,在Linux系统上可以使用OpenSSL库,需在代码开头添加以下代码:
```
#include <openssl/aes.h>
```
然后,定义一个函数来进行AES128加密:
```
int encrypt(unsigned char *plaintext, int plaintext_len, unsigned char *key, unsigned char *iv, unsigned char *ciphertext) {
AES_KEY aesKey;
if (AES_set_encrypt_key(key, 128, &aesKey) < 0) {
return -1;
}
AES_cbc_encrypt(plaintext, ciphertext, plaintext_len, &aesKey, iv, AES_ENCRYPT);
return 0;
}
```
在主函数中,可以调用上述函数进行加密:
```
int main() {
unsigned char *plaintext = (unsigned char *)"Hello, AES128!";
int plaintext_len = strlen((char *)plaintext);
unsigned char key[AES_BLOCK_SIZE];
memset(key, 0x00, sizeof(key)); // 这里的示例密钥全为0,实际使用时应使用随机生成的密钥
unsigned char iv[AES_BLOCK_SIZE];
memset(iv, 0x00, sizeof(iv)); // 这里的示例初始向量全为0,实际使用时应使用随机生成的初始向量
unsigned char ciphertext[plaintext_len];
memset(ciphertext, 0, sizeof(ciphertext));
if (encrypt(plaintext, plaintext_len, key, iv, ciphertext) != 0) {
printf("Encryption failed.\n");
return -1;
}
printf("Ciphertext: ");
for (int i = 0; i < plaintext_len; i++) {
printf("%02x", ciphertext[i]);
}
printf("\n");
return 0;
}
```
上述示例中,使用AES_set_encrypt_key函数设置密钥,使用AES_cbc_encrypt函数进行加密。其中,参数plaintext为待加密的数据,plaintext_len为数据的长度,key为AES128密钥,iv为初始向量,ciphertext为加密后的结果。
需要注意的是,实际应用中应该使用随机生成的密钥和初始向量,而不是示例中的全0值。此外,还需要进行错误处理和适当的内存管理,以保证程序的正确性和安全性。
### 回答3:
AES-128加密是一种对称加密算法,该算法使用128位的密钥对数据进行加密和解密运算。对于C语言来说,可以使用现有的密码库来实现AES-128加密。
一种常见的方法是使用OpenSSL库来实现AES加密。下面是一个使用AES-128-CBC模式加密和解密的示例代码:
```c
#include <stdio.h>
#include <openssl/aes.h>
void encrypt(const unsigned char *plaintext, int plaintext_len, const unsigned char *key, unsigned char *ciphertext) {
AES_KEY aes_key;
AES_set_encrypt_key(key, 128, &aes_key);
AES_encrypt(plaintext, ciphertext, &aes_key);
}
void decrypt(const unsigned char *ciphertext, int ciphertext_len, const unsigned char *key, unsigned char *plaintext) {
AES_KEY aes_key;
AES_set_decrypt_key(key, 128, &aes_key);
AES_decrypt(ciphertext, plaintext, &aes_key);
}
int main() {
const unsigned char plaintext[] = "Hello, World!";
const int plaintext_len = sizeof(plaintext) - 1;
const unsigned char key[] = "0123456789abcdef";
unsigned char ciphertext[plaintext_len];
encrypt(plaintext, plaintext_len, key, ciphertext);
printf("Ciphertext: ");
for (int i = 0; i < sizeof(ciphertext); i++) {
printf("%02x ", ciphertext[i]);
}
printf("\n");
unsigned char decrypted[plaintext_len];
decrypt(ciphertext, sizeof(ciphertext), key, decrypted);
printf("Decrypted: %s\n", decrypted);
return 0;
}
```
该代码中使用AES_set_encrypt_key函数和AES_encrypt函数进行加密操作,使用AES_set_decrypt_key函数和AES_decrypt函数进行解密操作。其中,plaintext是明文字符串,key是128位的密钥,ciphertext是加密后的密文,decrypted是解密后的明文。
需要注意的是,以上示例代码只是向你展示了如何使用OpenSSL库来进行AES-128加密,具体的应用场景和具体的实现方式还需要根据你的需求来确定。同时,使用加密算法时要注意密钥的安全性和合法性,并遵守相关法律法规。
阅读全文
相关推荐
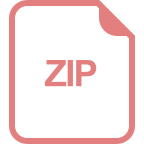

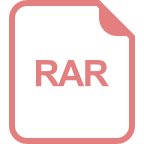
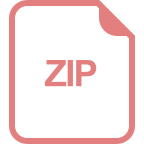
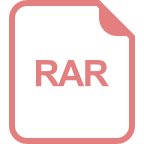
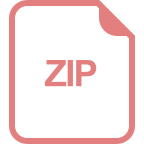
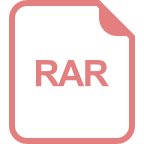
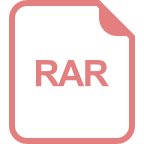

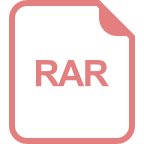
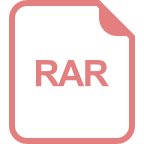
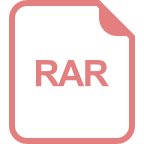
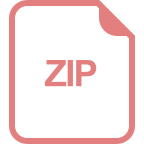

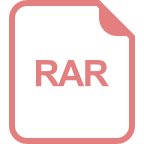