如何在C++中实现高精度的两个数相减并提供一个相关模板示例?
时间: 2024-12-31 12:47:34 浏览: 11
在C++中,实现高精度的两个数相减通常需要使用字符串或动态数组来存储大整数,因为标准库里的`int`或`long long`类型可能会受到数值范围的限制。可以创建一个自定义类,比如`BigInt`,用于表示大整数,并提供加、减等运算的方法。
下面是一个简单的模板类示例:
```cpp
#include <iostream>
#include <string>
template <typename CharT>
class BigInt {
public:
std::string value;
BigInt(const std::string& s) : value(s) {}
// 减法操作,假设这里我们只支持两个相同长度的大整数相减
BigInt operator-(const BigInt<CharT>& other) const {
if (value.size() > other.value.size()) {
std::swap(value, other.value);
}
BigInt result;
int carry = 0;
for (size_t i = 0; i < value.size(); ++i) {
int digit1 = value[i] - '0';
int digit2 = other.value[i] - '0';
int difference = digit1 - digit2 + carry;
carry = difference / 10;
result.value += static_cast<CharT>((difference % 10) + '0');
}
// 如果有负数结果,需要处理第一位的carry
if (carry > 0 || value.size() < other.value.size()) {
result.value.insert(0, 1, static_cast<CharT>(carry + '0'));
}
return result;
}
};
// 示例使用
int main() {
BigInt<char> num1("99999");
BigInt<char> num2("45678");
BigInt<char> diff = num1 - num2;
std::cout << "Difference: " << diff.value << std::endl;
return 0;
}
```
注意这个示例非常基础,实际应用中可能需要考虑更多的边缘情况和性能优化。另外,对于复杂的操作,如进位、借位,可能需要更复杂的算法和数据结构来实现。
阅读全文
相关推荐

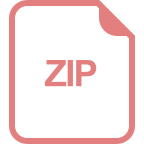
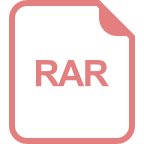















