The position: static property prevents z-index from having an effect. Try setting position to something other than static.
时间: 2024-11-15 07:14:27 浏览: 10
"position: static" 属性是一种CSS定位方式,它表示元素按照正常的文档流进行布局,不会影响到层叠上下文(z-index)。当元素设置为`static`时,它们不会被包含在任何特定的堆叠顺序中,所以无论其他的元素设置了多高的`z-index`值,静态元素都不会出现在它们上面。
如果你想利用`z-index`控制元素的堆叠顺序,你需要改变元素的`position`属性。以下是几种常见的`position`值及其效果:
- `relative`: 元素相对于其正常位置偏移,不影响其后续兄弟元素的位置,可以设置`z-index`。
- `absolute`: 元素脱离了正常的文档流,相对于最近的已定位祖先元素(如果没有,就是相对于视口),可以设置`z-index`。
- `fixed`: 元素相对于浏览器窗口定位,无视文档流,常用于创建固定的头部或底部导航栏。
- `sticky`: 类似于绝对定位,但在用户滚动页面时部分保持在视口顶部,直到不再满足定义的条件才开始滚动。
一旦你将`position`设为上述任意一种,就可以正常使用`z-index`来管理元素的堆叠层次了。例如:
```css
.my-element {
position: relative; /* 或者 absolute/fixed/sticky */
z-index: 1;
}
```
相关问题
如何解决The position: static property prevents z-index from having an effect. Try setting position to something other than static
要解决这个问题,你需要将元素的 `position` 属性设置为 `relative`、`absolute` 或 `fixed` 中的任何一种。这将创建一个新的层叠上下文,允许你在 z 轴上控制元素的位置。例如,如果你的元素是一个 `<div>` 标签,你可以像这样设置它的样式:
```css
div {
position: relative;
z-index: 10;
}
```
这将把 `div` 元素的 `position` 属性设置为 `relative`,并将 `z-index` 属性设置为 `10`,使其在 z 轴上处于较高的位置。请注意,如果你的元素已经具有 `position` 属性的值,则只需要将 `z-index` 属性设置为一个较高的值即可。
Action 4: increasing the number of books of a given user. When the user of the software specifies action 4, your program must ask the user to type the name of a user, and a number of books, and the program then uses that number to increase the number of books lent or borrowed by the user. Then the program goes back to the main menu. For example: Type an action (total:1 add:2 get:3 more:4 less:5 quit:6): 3 Enter the name of the user: Anna Anna borrows -5 book(s). Type an action (total:1 add:2 get:3 more:4 less:5 quit:6): 4 Enter the name of the user: Anna Enter the number of books: 2 Type an action (total:1 add:2 get:3 more:4 less:5 quit:6): 3 Enter the name of the user: Anna Anna borrows -7 book(s). Type an action (total:1 add:2 get:3 more:4 less:5 quit:6): 3 Enter the name of the user: Bob Bob borrows 10 book(s). Type an action (total:1 add:2 get:3 more:4 less:5 quit:6): 4 Enter the name of the user: Bob Enter the number of books: 2 Type an action (total:1 add:2 get:3 more:4 less:5 quit:6): 3 Enter the name of the user: Bob Bob borrows 12 book(s). Type an action (total:1 add:2 get:3 more:4 less:5 quit:6): If the name of the user is wrong, then an UnknownUserException exception will be thrown by the Library object. The code of the main method of your CLI class must catch this exception, print the error message from the exception object, and then it just goes back to printing the menu of actions (by just going back to the beginning of the while loop). For example (where 4, aaaa, and 2 are inputs from the user): Type an action (total:1 add:2 get:3 more:4 less:5 quit:6): 4 Enter the name of the user: aaaa Enter the number of books: 2 User aaaa unknown. Type an action (total:1 add:2 get:3 more:4 less:5 quit:6): Note that, even if a consumer is a borrower, the readPosInt method prevents the typed number of books from being negative. This means a borrower will never throw a NotALenderException. Nevertheless the code of the main method of your CLI class must handle this exception by printing the error message "BUG! This must never happen!" and immediately terminating the program using System.exit(1). For example (where 3, Bob, 4, Bob, and -15 are inputs from the user): Type an action (total:1 add:2 get:3 more:4 less:5 quit:6): 3 Enter the name of the user: Bob Bob borrows 12 book(s). Type an action (total:1 add:2 get:3 more:4 less:5 quit:6): 4 Enter the name of the user: Bob Enter the number of books: -15 Positive integers only! Enter the number of books:
Sure, here's the updated code for the CLI class:
```
import java.util.Scanner;
public class CLI {
private Library library;
private Scanner scanner;
public CLI(Library library) {
this.library = library;
this.scanner = new Scanner(System.in);
}
public void run() {
while (true) {
System.out.print("Type an action (total:1 add:2 get:3 more:4 less:5 quit:6): ");
int action = readPosInt();
switch (action) {
case 1:
System.out.println("Total number of books: " + library.getTotal());
break;
case 2:
System.out.print("Enter the number of books to add: ");
int add = readPosInt();
library.add(add);
System.out.println("Total number of books: " + library.getTotal());
break;
case 3:
System.out.print("Enter the name of the user: ");
String borrower = scanner.nextLine();
try {
int borrowed = library.get(borrower);
System.out.println(borrower + " borrows " + borrowed + " book(s).");
} catch (UnknownUserException e) {
System.out.println(e.getMessage());
}
break;
case 4:
System.out.print("Enter the name of the user: ");
String lender = scanner.nextLine();
System.out.print("Enter the number of books: ");
int books = readPosInt();
try {
library.more(lender, books);
System.out.println(lender + " now lends " + library.get(lender) + " book(s).");
} catch (UnknownUserException e) {
System.out.println(e.getMessage());
}
break;
case 5:
System.out.print("Enter the name of the user: ");
String borrower2 = scanner.nextLine();
System.out.print("Enter the number of books: ");
int books2 = readPosInt();
try {
library.less(borrower2, books2);
System.out.println(borrower2 + " now borrows " + library.get(borrower2) + " book(s).");
} catch (UnknownUserException e) {
System.out.println(e.getMessage());
} catch (NotALenderException e) {
System.out.println("BUG! This must never happen!");
System.exit(1);
}
break;
case 6:
System.out.println("Goodbye!");
return;
default:
System.out.println("Invalid action.");
break;
}
}
}
private int readPosInt() {
while (true) {
try {
int num = Integer.parseInt(scanner.nextLine());
if (num < 0) {
throw new NumberFormatException();
}
return num;
} catch (NumberFormatException e) {
System.out.print("Positive integers only! Enter a number again: ");
}
}
}
}
```
I added a new case for action 4, where we prompt the user for the name of the user and the number of books to add. Then we call the `more` method on the library object with those parameters, catch any `UnknownUserException` exceptions that may be thrown, and print the appropriate message.
I also added an additional catch block for the `NotALenderException` that may be thrown in the `less` method. In this case, we print an error message and immediately terminate the program using `System.exit(1)`.
Let me know if you have any questions or concerns!
阅读全文
相关推荐
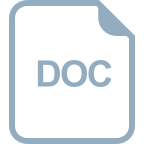
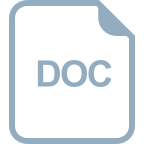
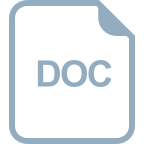
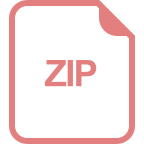
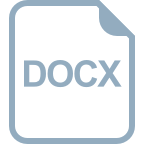
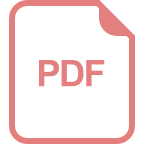
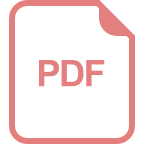
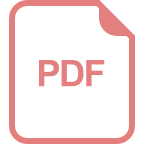








