用C++编写对本地文件的编码与解码
时间: 2024-10-18 16:08:38 浏览: 18
在C++中,处理文件的编码和解码通常涉及到标准库中的`locale`、`codecvt`和`fstream`等组件。以下是基本步骤:
1. **包含头文件**:
```cpp
#include <iostream>
#include <fstream>
#include <locale>
#include <codecvt> // 引入字符转换类别
```
2. **设置当前locale**:
使用`std::locale`可以指定特定的语言环境,这会影响文件读写的编码规则。
```cpp
std::locale loc("your_locale"); // "your_locale"应替换为你所需的编码,如"zh_CN.UTF-8"
```
3. **打开文件流**:
```cpp
std::ifstream file("path_to_your_file", std::ios::in | std::ios::binary); // 使用二进制模式
std::ofstream out("path_to_output_file", std::ios::out | std::ios::binary);
```
4. **选择编码**:
C++没有直接支持所有编码格式,你可以使用第三方库如ICU (International Components for Unicode) 或 Boost.Locale 来处理。如果文件已知是UTF-8,可以直接读取。
5. **读取和写入**:
```cpp
std::string buffer;
file.imbue(loc); // 将locale应用到流上
file.read(buffer.data(), buffer.size()); // 读取文件内容
// 解码(假设是UTF-8)
if (file.gcount() > 0) {
auto decoded = std::wstring_convert<std::codecvt_utf8<char16_t>, char16_t>()
.from_bytes(buffer);
// 这里做进一步处理,如打印或保存到其他编码格式
}
// 对于写入,先将字符串转换成目标编码
if (auto encoded = ...; // 编码操作,例如从wstring to UTF-8
!encoded.empty()) {
out << encoded;
out.flush();
}
```
6. **关闭文件**:
```cpp
file.close();
out.close();
```
阅读全文
相关推荐
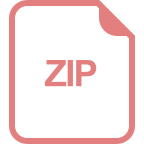
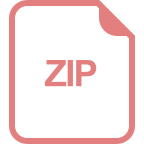
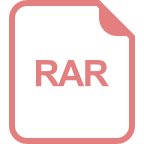















