numpy能实现n维向量叉乘吗
时间: 2024-12-18 16:28:55 浏览: 14
在NumPy库中,虽然它主要用于处理数组操作,但它并不直接提供内置函数来计算二维或更高维度的向量叉乘(也称为向量积)。二维空间下的两个向量可以使用`numpy.cross()`函数进行交叉相乘运算,但这只适用于二维情况,对于更高维度的向量,通常需要先降维到三维再进行交叉。
如果你有一个更高维度的向量,并希望对其进行类似的操作,可能需要将其展平为三维,然后利用`numpy.cross()`,最后再将结果重塑回原始形状。例如:
```python
import numpy as np
def cross_product_nd(vector):
# 将高维向量展平为三维
vector_3d = np.reshape(vector, (-1, 1, len(vector)))
# 计算交叉积
result = np.cross(vector_3d[0], vector_3d[1])
# 返回结果并重塑回原形状
return result.reshape(*vector.shape[:-1], -1)
# 使用示例
vector = np.array([[[1, 2, 3]], [[4, 5, 6]]]) # 一个二阶的三通道向量
cross_result = cross_product_nd(vector)
```
请注意,这并不是标准的NumPy做法,因为通常在高维空间中,向量之间的“交”操作是没有意义的,除非在特定上下文中(比如物理学中的矢量张量运算)。
相关问题
用phthon编写一个实现n维向量各种操作的类,主要功能包括: (1) 编写成员函数求两个向量的内积; (2) 编写成员函数求两个向量的外积; (3) 编写成员函数求两个向量的和; (4) 编写成员函数求两个向量的差; (5) 在图形界面类上编写一个测试该类的程序。
Python 中可以创建一个名为 `Vector` 的类,用于实现 n 维向量的各种操作:
```python
import numpy as np
class Vector:
def __init__(self, components):
self.components = np.array(components)
# 成员函数:计算内积
def dot_product(self, other):
if len(self.components) != len(other.components):
raise ValueError("Vectors must have the same dimension for inner product.")
return np.dot(self.components, other.components)
# 成员函数:计算外积(叉乘),适用于二维向量
def cross_product(self, other):
if len(self.components) != 2 or len(other.components) != 2:
raise ValueError("Cross product is only applicable to two-dimensional vectors.")
x = self.components[1] * other.components[0]
y = -self.components[0] * other.components[1]
return Vector([x, y])
# 成员函数:向量加法
def add(self, other):
return Vector(self.components + other.components)
# 成员函数:向量减法
def subtract(self, other):
return Vector(self.components - other.components)
# 测试类
def test_vector_operations():
v1 = Vector([1, 2, 3])
v2 = Vector([4, 5, 6])
print(f"Inner product: {v1.dot_product(v2)}")
print(f"Cross product (if 2D): {v1.cross_product(v2).components if len(v1.components) == 2 else 'Not available'}")
print(f"Sum: {v1.add(v2).components}")
print(f"Difference: {v1.subtract(v2).components}")
# 执行测试
test_vector_operations()
```
在这个示例中,我们首先导入了 NumPy 库,因为它提供方便的向量处理功能。然后定义了一个 `Vector` 类,包含了五个成员函数。注意,对于二维向量的外积(叉乘),我们只实现了二元操作,并在代码中进行了条件检查。
最后,我们定义了一个 `test_vector_operations` 函数,用于创建两个向量并展示各项操作的结果。在实际的 GUI 程序中,你可以将这个测试部分包装到图形用户界面中,让用户输入向量并触发相应的操作。
半程向量与法向量的角度差代码
假设我们有一个平面或者一个三维空间中的三角形,其三个顶点分别为 $A(x_1, y_1, z_1)$,$B(x_2, y_2, z_2)$,$C(x_3, y_3, z_3)$。
首先,我们可以通过向量的减法计算出两个边的向量:
$$\vec{AB} = \begin{pmatrix}x_2 - x_1 \\ y_2 - y_1 \\ z_2 - z_1\end{pmatrix} \quad \vec{AC} = \begin{pmatrix}x_3 - x_1 \\ y_3 - y_1 \\ z_3 - z_1\end{pmatrix}$$
接着,我们可以通过向量的叉乘计算出该三角形的法向量:
$$\vec{n} = \vec{AB} \times \vec{AC}$$
最后,我们可以通过余弦定理计算出半程向量和法向量之间的夹角:
$$\cos \theta = \frac{\vec{AB} \cdot \vec{n}}{|\vec{AB}| \cdot |\vec{n}|}$$
其中,$\cdot$ 表示向量的点乘,$|\vec{v}|$ 表示向量 $\vec{v}$ 的模长。因此,代码实现如下:
```python
import numpy as np
def angle_between_halfvector_and_normalvector(A, B, C):
AB = np.array(B) - np.array(A)
AC = np.array(C) - np.array(A)
normal_vector = np.cross(AB, AC)
half_vector = -AB / np.linalg.norm(AB)
cos_theta = np.dot(half_vector, normal_vector) / (np.linalg.norm(half_vector) * np.linalg.norm(normal_vector))
return np.arccos(cos_theta)
```
其中,`A`、`B`、`C` 表示三角形的三个顶点坐标,函数返回半程向量和法向量之间的夹角(弧度制)。
阅读全文
相关推荐
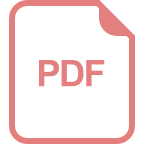
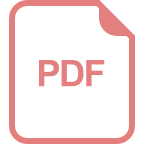
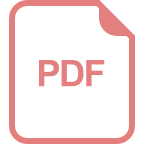
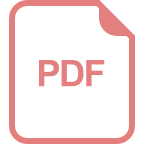
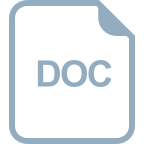
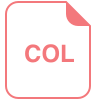
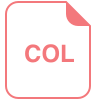
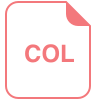
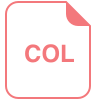
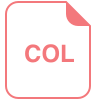
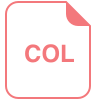
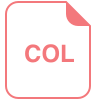
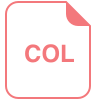


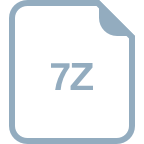
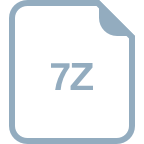