树莓派mpu6050滤波算法实现代码
时间: 2024-06-19 07:03:41 浏览: 296
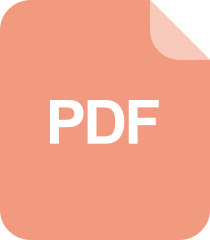
mpu6050滤波算法
树莓派的MPU6050滤波算法有多种实现方式,其中一种比较常用的是卡尔曼滤波算法。以下是一个基于Python的树莓派MPU6050卡尔曼滤波算法实现的示例代码:
```
import math
import time
import smbus
bus = smbus.SMBus(1)
address = 0x68
def read_byte(reg):
return bus.read_byte_data(address, reg)
def read_word(reg):
high = bus.read_byte_data(address, reg)
low = bus.read_byte_data(address, reg+1)
value = (high << 8) + low
return value
def read_word_2c(reg):
val = read_word(reg)
if (val >= 0x8000):
return -((65535 - val) + 1)
else:
return val
def dist(a,b):
return math.sqrt((a*a)+(b*b))
def get_y_rotation(x,y,z):
radians = math.atan2(x, dist(y,z))
return -math.degrees(radians)
def get_x_rotation(x,y,z):
radians = math.atan2(y, dist(x,z))
return math.degrees(radians)
def kalman_filter(x, P, Q, R, measurement):
K = P / (P + R)
x = x + K * (measurement - x)
P = (1 - K) * P + Q
return x, P
def main():
# MPU6050 registers
power_mgmt_1 = 0x6b
power_mgmt_2 = 0x6c
# Wake up the MPU6050
bus.write_byte_data(address, power_mgmt_1, 0)
# Kalman filter variables
kalman_x = 0
kalman_y = 0
kalman_x_P = 1
kalman_y_P = 1
kalman_Q = 0.00001
kalman_R = 0.01
while True:
gyro_xout = read_word_2c(0x43)
gyro_yout = read_word_2c(0x45)
gyro_zout = read_word_2c(0x47)
accel_xout = read_word_2c(0x3b)
accel_yout = read_word_2c(0x3d)
accel_zout = read_word_2c(0x3f)
accel_xout_scaled = accel_xout / 16384.0
accel_yout_scaled = accel_yout / 16384.0
accel_zout_scaled = accel_zout / 16384.0
gyro_xout_scaled = gyro_xout / 131.0
gyro_yout_scaled = gyro_yout / 131.0
gyro_zout_scaled = gyro_zout / 131.0
# Filter the accelerometer data using the Kalman filter
kalman_x, kalman_x_P = kalman_filter(kalman_x, kalman_x_P, kalman_Q, kalman_R, accel_xout_scaled)
kalman_y, kalman_y_P = kalman_filter(kalman_y, kalman_y_P, kalman_Q, kalman_R, accel_yout_scaled)
# Compute the roll and pitch angles using the filtered accelerometer data
roll_angle = get_x_rotation(accel_xout_scaled, accel_yout_scaled, accel_zout_scaled)
pitch_angle = get_y_rotation(accel_xout_scaled, accel_yout_scaled, accel_zout_scaled)
# Compute the yaw angle using the gyro data
yaw_angle = gyro_zout_scaled * 0.98
# Print the roll, pitch, and yaw angles
print("Roll: {:.2f} degrees".format(roll_angle))
print("Pitch: {:.2f} degrees".format(pitch_angle))
print("Yaw: {:.2f} degrees".format(yaw_angle))
# Delay for a short period of time
time.sleep(0.1)
if __name__ == "__main__":
main()
```
该示例代码使用了卡尔曼滤波算法对MPU6050传感器获取的加速度计数据进行了滤波,并计算出了姿态角(roll、pitch、yaw)。你可以根据自己的需求进行修改和优化。如果你对树莓派和MPU6050还有其他问题,欢迎提出。
阅读全文
相关推荐
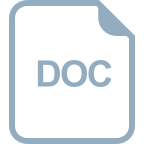
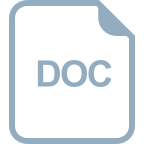
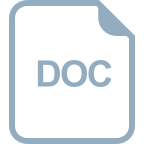
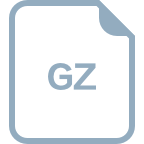
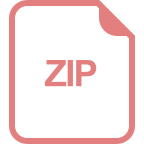
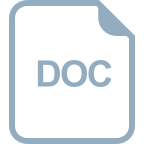
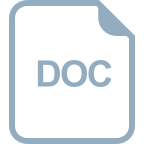
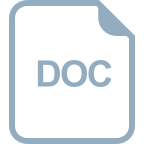
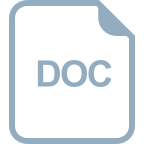


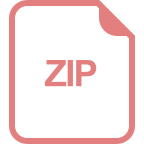
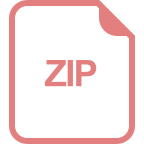