随机梯度下降L2正则化 python代码
时间: 2023-11-16 08:58:16 浏览: 53
下面是使用随机梯度下降和L2正则化的Python代码实现:
```
import numpy as np
from sklearn.linear_model import SGDRegressor
X = 2*np.random.rand(100,1)
y = 4 + 3*X + np.random.randn(100, 1)
# 使用随机梯度下降的方法,penalty代表使用哪种正则项,alpha代表正则化强度,max_iter代表迭代多少次
sgd_reg = SGDRegressor(penalty='l2', alpha=0.1, max_iter=1000)
sgd_reg.fit(X, y.reshape(-1,))
print(sgd_reg.predict([[1.5]]))
print(sgd_reg.intercept_)
print(sgd_reg.coef_)
```
其中,`penalty='l2'`表示使用L2正则化,`alpha`表示正则化强度,`max_iter`表示迭代次数。`fit`方法用于训练模型,`predict`方法用于预测新的数据。
相关问题
用梯度下降算法实现Logistic回归的L1正则化和L2正则化python代码
下面是用梯度下降算法实现Logistic回归的L1正则化和L2正则化的Python代码:
```python
import numpy as np
class LogisticRegression:
def __init__(self, lr=0.1, num_iter=1000, fit_intercept=True, regularization=None, lambda_=0.1):
self.lr = lr
self.num_iter = num_iter
self.fit_intercept = fit_intercept
self.regularization = regularization
self.lambda_ = lambda_
def __add_intercept(self, X):
intercept = np.ones((X.shape[0], 1))
return np.concatenate((intercept, X), axis=1)
def __sigmoid(self, z):
return 1 / (1 + np.exp(-z))
def __loss(self, h, y):
return (-y * np.log(h) - (1 - y) * np.log(1 - h)).mean()
def __l1_regularization(self, w):
return self.lambda_ * np.abs(w[1:]).sum()
def __l2_regularization(self, w):
return self.lambda_ * np.sum(w[1:] ** 2)
def fit(self, X, y):
if self.fit_intercept:
X = self.__add_intercept(X)
self.theta = np.zeros(X.shape[1])
for i in range(self.num_iter):
z = np.dot(X, self.theta)
h = self.__sigmoid(z)
if self.regularization == 'l1':
# L1正则化
grad = np.dot(X.T, (h - y)) / y.size + self.lambda_ * np.sign(self.theta)
elif self.regularization == 'l2':
# L2正则化
grad = np.dot(X.T, (h - y)) / y.size + self.lambda_ * self.theta
else:
grad = np.dot(X.T, (h - y)) / y.size
self.theta -= self.lr * grad
def predict_prob(self, X):
if self.fit_intercept:
X = self.__add_intercept(X)
return self.__sigmoid(np.dot(X, self.theta))
def predict(self, X, threshold=0.5):
return self.predict_prob(X) >= threshold
```
其中,lr是学习率,num_iter是迭代次数,fit_intercept表示是否拟合截距,regularization表示正则化方法,lambda_是正则化系数。在fit方法中,通过判断regularization的取值,来实现L1正则化和L2正则化。在L1正则化中,使用np.sign函数计算符号函数,而在L2正则化中,直接对参数的平方和进行惩罚。在predict_prob方法中,对X进行截距拟合和sigmoid变换,返回预测概率。在predict方法中,对预测概率进行阈值处理,返回预测结果。
随机森林与l2正则化混合代码实例
以下是使用随机森林和L2正则化进行混合的Python代码示例:
```python
from sklearn.ensemble import RandomForestClassifier
from sklearn.linear_model import LogisticRegression
from sklearn.pipeline import make_pipeline
from sklearn.preprocessing import StandardScaler
# 创建随机森林模型
rf = RandomForestClassifier(n_estimators=100, random_state=42)
# 创建逻辑回归模型
lr = LogisticRegression(penalty='l2', random_state=42)
# 创建管道,先进行特征标准化,再使用随机森林和逻辑回归模型进行混合
pipeline = make_pipeline(StandardScaler(), rf, lr)
# 使用管道进行训练和预测
pipeline.fit(X_train, y_train)
y_pred = pipeline.predict(X_test)
```
在上述代码中,我们首先使用随机森林和逻辑回归模型分别创建了`rf`和`lr`对象。然后,我们使用`make_pipeline`函数创建了一个管道,该管道首先对特征进行标准化,然后使用随机森林和逻辑回归模型进行混合。最后,我们使用管道进行训练和预测。
需要注意的是,L2正则化的超参数C可以通过`LogisticRegression`的`C`参数进行设置。此外,还可以使用其他正则化方法,例如L1正则化或弹性网络正则化,以及其他集成方法,例如梯度提升树等。
相关推荐
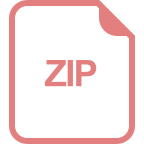
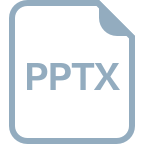
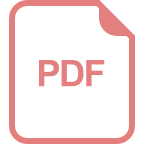
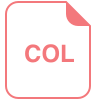
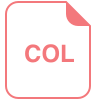
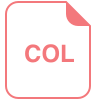
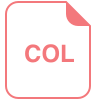
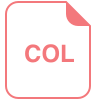







