怎么遍历线索二叉树提供代码和输出案例
时间: 2024-09-18 09:06:02 浏览: 31
线索二叉树是一种特殊的二叉搜索树,它除了存储节点值外,还额外保存了一些指向前驱和后继节点的线索信息。遍历线索二叉树通常有三种常见的方式:前序遍历、中序遍历和后序遍历。
**1. 前序遍历(先根遍历):**
```python
def in_order_traversal_with_links(root):
if root is not None:
# 先访问当前节点
print(root.val)
# 然后访问左子树
in_order_traversal_with_links(root.left)
# 最后访问右子树
in_order_traversal_with_links(root.right)
```
**2. 中序遍历:**
```python
def in_order_traversal_with_links(root):
if root is not None:
in_order_traversal_with_links(root.left)
# 中间访问当前节点
print(root.val)
in_order_traversal_with_links(root.right)
```
**3. 后序遍历:**
```python
def post_order_traversal_with_links(root):
if root is not None:
post_order_traversal_with_links(root.left)
post_order_traversal_with_links(root.right)
# 最后访问当前节点
print(root.val)
```
请注意,这里假设`root`是一个包含`val`, `left`, 和`right`属性的节点,并且`left`和`right`属性分别指向其左孩子和右孩子。如果线索保存在额外的`prev`和`next`字段中,则需要相应地调整递归调用。
**案例输出:**
例如,对于线索二叉树:
```
5
/ \
3 8
/ \ / \
1 4 6 9
```
前序遍历会输出:5, 3, 1, 4, 8, 6, 9
中序遍历会输出:1, 3, 4, 5, 6, 8, 9
后序遍历会输出:1, 4, 3, 6, 9, 8, 5
阅读全文
相关推荐
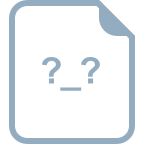
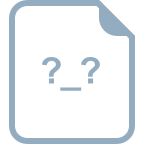
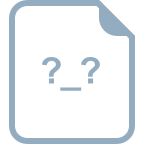
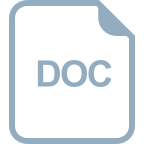
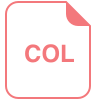
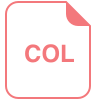
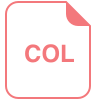
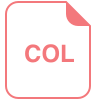
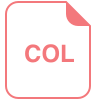
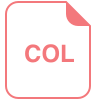
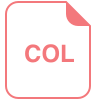
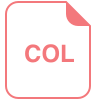
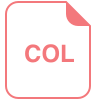
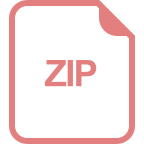
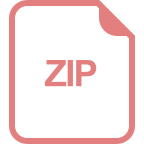
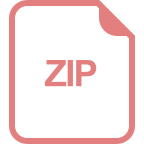
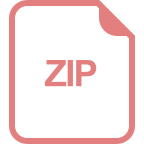