yolov9在代码中导入yolo模块
时间: 2024-08-12 10:01:33 浏览: 107
在使用Ultralytics YOLOv9的Python代码中,导入`yolo`模块通常是在`__init__.py`或者直接在需要用到它的地方进行的。以下是导入的基本示例:
```python
# 如果你在项目目录下有一个名为ultralytics的子目录,其中包含yolo模块
from .yolo import *
# 或者如果yolo.py文件就在当前目录下
import yolo
# 初始化模型和配置
yolo_model = YOLOv9()
config = Config("path/to/custom_config.yaml") # 替换为你的配置文件路径
# 开始推理
results = yolo_model.predict(image_path) # image_path是你想要检测图片的路径
```
注意替换`"path/to/custom_config.yaml"`为你实际的配置文件路径,以及根据你的需求选择合适的模型初始化方式(可能是默认模型,或者是加载自定义训练的权重)。
相关问题
yolov5代码详解yolo.py
yolov5是一个目标检测算法,yolo.py是其中的一个核心文件,主要实现了模型的构建和训练。下面是yolo.py的代码详解:
1. 导入必要的库和模块
```python
import torch
import torch.nn as nn
import numpy as np
from collections import OrderedDict
from utils.general import anchors, autopad, scale_img, check_anchor_order, check_file, check_img_size, \
check_requirements, non_max_suppression, xyxy2xywh, xywh2xyxy, plot_one_box
from utils.torch_utils import time_synchronized, fuse_conv_and_bn, model_info
from models.common import Conv, DWConv
```
2. 定义YOLOv5模型
```python
class YOLOv5(nn.Module):
def __init__(self, nc=80, anchors=(), ch=(), inference=False): # model, input channels, number of classes
super(YOLOv5, self).__init__()
self.nc = nc # number of classes
self.no = nc + 5 # number of outputs per anchor
self.nl = len(anchors) # number of detection layers
self.na = len(anchors[0]) // 2 # number of anchors per layer
self.grid = [torch.zeros(1)] * self.nl # init grid
a = torch.tensor(anchors).float().view(self.nl, -1, 2)
self.register_buffer('anchors', a) # shape(nl,na,2)
self.register_buffer('anchor_grid', a.clone().view(self.nl, 1, -1, 1, 1, 2)) # shape(nl,1,na,1,1,2)
self.m = nn.ModuleList(nn.Conv2d(x, self.no * self.na, 1) for x in ch) # output conv
self.inference = inference # inference flag
```
3. 定义前向传播函数
```python
def forward(self, x):
self.img_size = x.shape[-2:] # store image size
x = self.forward_backbone(x) # backbone
z = [] # inference output
for i in range(self.nl):
x[i] = self.m[i](x[i]) # conv
bs, _, ny, nx = x[i].shape # x(bs,255,20,20) to x(bs,3,20,20,85)
x[i] = x[i].view(bs, self.na, self.no, ny, nx).permute(0, 1, 3, 4, 2).contiguous()
if not self.training: # inference
if self.inference == 'tflite':
z.append(x[i].detach().cpu()) # inference tflite
else:
io = x[i].sigmoid()
io[..., 4:] = io[..., 4:] * io[..., 4:].mean(1, keepdim=True) * self.nc # sigmoid obj,class scores
bxy = io[..., :2].sigmoid() * 2. - 0.5 + self.grid[i] # xy
bwh = io[..., 2:4].exp() * self.anchor_grid[i] # wh
xywh = torch.cat((bxy, bwh), -1).view(bs, -1, 4) * self.stride[i] # xywh (center+offset) relative to image size
z.append(xywh.view(bs, -1, self.no), ) # xywhn
return x if self.training else (torch.cat(z, 1), x)
```
4. 定义后向传播函数
```python
def forward_backbone(self, x):
x = self.conv1(x)
x = self.bn1(x)
x = self.act1(x)
x = self.pool1(x)
x = self.layer1(x)
x = self.layer2(x)
x = self.layer3(x)
x = self.layer4(x)
x = self.layer5(x)
x = self.layer6(x)
x = self.layer7(x)
x = self.layer8(x)
x = self.layer9(x)
return x
```
以上就是yolo.py的代码详解,其中包括了YOLOv5模型的定义和前向传播函数的实现。相关问题如下:
相关问题:
1. YOLOv5模型的输入和输出是什么?
2. YOLOv5模型的训练过程是怎样的?
3. YOLOv5模型中的anchors是什么?
yolov8 pose 转 yolo
对于将YOLOv8 Pose转换为YOLO格式,可以按照以下步骤进行:
1. 首先,确保您已经安装了ultralytics库。如果没有安装,可以使用pip来安装ultralytics库。
2. 创建一个新的脚本,并导入ultralytics库的YOLO模块。
```python
from ultralytics import YOLO
```
3. 初始化YOLOv8 Pose模型。使用YOLO函数并传入YOLOv8-s Pose的权重文件路径。
```python
model = YOLO("yolov8s-pose.pt")
```
4. 使用export方法将模型转换为YOLO格式的onnx文件。设置format参数为"onnx",simplify参数为True以简化模型。
```python
success = model.export(format="onnx", simplify=True)
```
5. 确认模型成功转换为YOLO格式的onnx文件。
```python
assert success
print("转换成功")
```
通过以上步骤,您可以将YOLOv8 Pose模型转换为YOLO格式。请确保在转换过程中按照正确的路径和文件名指定模型的位置。<span class="em">1</span><span class="em">2</span><span class="em">3</span><span class="em">4</span>
阅读全文
相关推荐
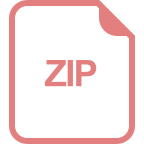
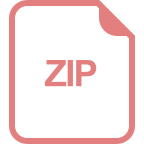
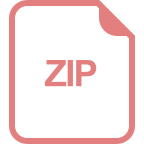













