python删除子字符串
时间: 2024-09-22 07:05:04 浏览: 55
在Python中,你可以使用`str.replace()`函数或`str.remove()`方法来删除子字符串。这里有两个例子:
1. `replace()`函数:如果你想要替换所有出现的子字符串,而不是只删除第一个,可以这样做:
```python
original_string = "Hello, World!"
substring_to_remove = "World"
new_string = original_string.replace(substring_to_remove, "")
print(new_string) # 输出: "Hello, !"
```
2. `remove()`方法:这个方法会移除字符串中首次出现的指定子字符串,如果子字符串不存在则不会有任何影响:
```python
original_string = "Hello, World!"
substring_to_remove = "World"
if substring_to_remove in original_string:
new_string = original_string.replace(substring_to_remove, "")
else:
new_string = original_string
print(new_string) # 输出: "Hello, !", 如果"World"不在原字符串中,则保持不变
```
请注意,`remove()`方法不会返回新字符串,而是直接修改原始字符串。
相关问题
Python删除子字符串,例如字符串s为abcabcd,子串c为bc,则调用该函数后,结果字符串s为aad。
在Python中,你可以使用`replace()`函数或者切片操作来删除子字符串。这里提供两个方法:
1. 使用 `replace()` 函数:
```python
def remove_substring(s, substr):
s = s.replace(substr, '')
return s
s = "abcabcd"
substr = "bc"
result = remove_substring(s, substr)
print(result) # 输出: "aadd"
```
在这个例子中,函数将字符串`s`中的子串"bc"替换为空字符串,达到删除的效果。
2. 切片操作:
```python
def remove_substring_by_slice(s, substr):
start_index = s.index(substr)
end_index = start_index + len(substr)
s = s[:start_index] + s[end_index:]
return s
result = remove_substring_by_slice(s, substr)
print(result) # 输出: "aadd"
```
这里首先找到子串在原字符串中的起始位置,然后取掉这个子串的部分,保留剩余部分。
python mysql 删除 字符串包含特定字符串的字段
可以使用 LIKE 运算符结合 % 或 _ 通配符来删除包含特定字符串的字段。
下面是一个例子:
```python
import mysql.connector
# 连接数据库
mydb = mysql.connector.connect(
host="localhost",
user="yourusername",
password="yourpassword",
database="mydatabase"
)
# 创建游标
mycursor = mydb.cursor()
# 删除包含特定字符串的字段
sql = "DELETE FROM customers WHERE address LIKE '%Lane%'"
mycursor.execute(sql)
# 提交更改
mydb.commit()
print(mycursor.rowcount, "记录被删除")
```
这个例子中,我们使用了 LIKE 运算符和 % 通配符来匹配包含 "Lane" 子字符串的 address 字段。然后我们执行 DELETE 语句将符合条件的记录删除。最后我们提交更改并打印出受影响的记录数。
阅读全文
相关推荐
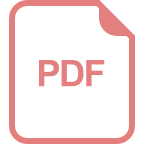
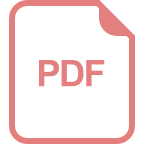
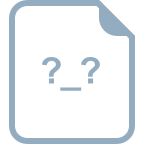













