ga-pso的代码python
时间: 2024-02-02 08:01:36 浏览: 294
ga-pso是遗传算法和粒子群优化算法的结合,它综合了两种算法的优点,使得优化的结果更加稳定和高效。
以下是一个简单的用Python实现ga-pso的代码示例:
```python
import numpy as np
# 设置参数
population_size = 50
max_iter = 100
c1 = 2
c2 = 2
w = 0.5
max_velocity = 0.2
# 初始化种群
def initialize_population(population_size):
population = []
for _ in range(population_size):
position = np.random.uniform(-5, 5, 2)
velocity = np.zeros(2)
pbest_position = position
pbest_value = fitness_function(position)
particle = {'position': position, 'velocity': velocity, 'pbest_position': pbest_position, 'pbest_value': pbest_value}
population.append(particle)
return population
# 适应度函数
def fitness_function(x):
return np.sum(x**2)
# 更新粒子速度和位置
def update_particle(particle, gbest_position):
new_velocity = w * particle['velocity'] + c1 * np.random.rand() * (particle['pbest_position'] - particle['position']) + c2 * np.random.rand() * (gbest_position - particle['position'])
new_velocity = np.clip(new_velocity, -max_velocity, max_velocity)
new_position = particle['position'] + new_velocity
new_value = fitness_function(new_position)
if new_value < particle['pbest_value']:
particle['pbest_position'] = new_position
particle['pbest_value'] = new_value
particle['position'] = new_position
particle['velocity'] = new_velocity
# 主函数
def ga_pso(population_size, max_iter):
population = initialize_population(population_size)
gbest_position = population[0]['position']
gbest_value = fitness_function(gbest_position)
for _ in range(max_iter):
for particle in population:
if particle['pbest_value'] < gbest_value:
gbest_position = particle['pbest_position']
gbest_value = particle['pbest_value']
update_particle(particle, gbest_position)
return gbest_position, gbest_value
# 运行算法
best_position, best_value = ga_pso(population_size, max_iter)
print('最优解:', best_position)
print('最优值:', best_value)
```
这是一个简单的用Python实现的ga-pso算法示例。在这个示例中,我们设置了种群大小为50,最大迭代次数为100,粒子的速度和位置更新规则使用了惯性权重w、加速系数c1和c2等参数。通过运行算法,我们可以得到一个优化问题的最优解和最优值。这个示例可以作为ga-pso算法的起点,根据具体的优化问题进行调整和改进。
阅读全文
相关推荐
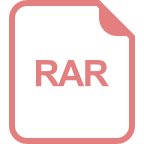

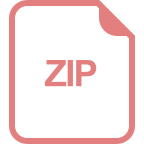
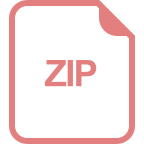

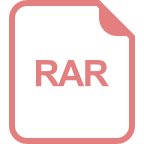
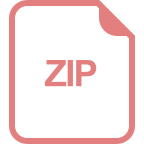
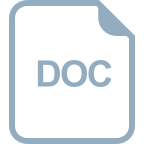
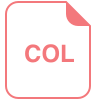
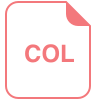
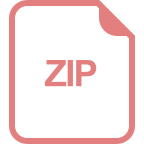
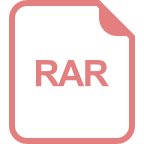
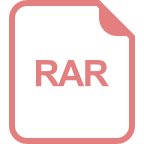
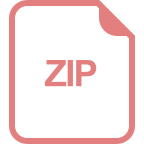
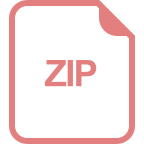
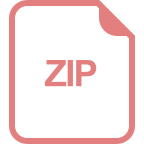
