java中我遇到一个问题如下,定义一个图形接口Shape,包含一个求面积的抽象方法。. 定义圆形(Circle)和长方形(Rectangle)实现图形接口。 实例化圆形和长方形的对象,并输出面积(利用多态的思想)。帮我解决一下
时间: 2024-11-24 08:42:14 浏览: 14
在 Java 中,你可以按照以下步骤使用多态来解决这个问题:
首先,定义一个名为 `Shape` 的接口,它有一个抽象方法 `getArea()` 表示计算面积的行为:
```java
public interface Shape {
double getArea();
}
```
接着,分别创建两个实现了 `Shape` 接口的类,如 `Circle` 和 `Rectangle`:
```java
public class Circle implements Shape {
private double radius;
public Circle(double radius) {
this.radius = radius;
}
@Override
public double getArea() {
return Math.PI * radius * radius;
}
}
public class Rectangle implements Shape {
private double length;
private double width;
public Rectangle(double length, double width) {
this.length = length;
this.width = width;
}
@Override
public double getArea() {
return length * width;
}
}
```
然后,在主程序中,你可以创建这两个类的具体实例,并通过它们共享 `Shape` 接口来调用 `getArea()` 方法,展示多态性:
```java
public class Main {
public static void main(String[] args) {
Shape circle = new Circle(5);
Shape rectangle = new Rectangle(4, 6);
System.out.println("Circle area: " + circle.getArea());
System.out.println("Rectangle area: " + rectangle.getArea());
// 这里就是多态的应用,circle和rectangle都是Shape类型的引用,但是运行时会根据实际对象的类型调用各自的getArea方法
}
}
```
当你运行这段代码时,将分别打印出圆形和矩形的面积。
阅读全文
相关推荐
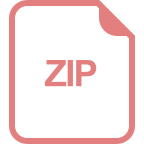
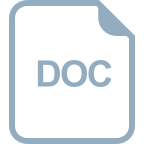
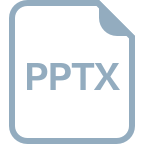
















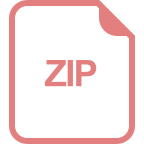