ModelBin read index_array failed 3361 layer load_model 16 /backbone/features.3/conv/conv.6/Conv failed
时间: 2024-01-01 22:04:32 浏览: 112
抱歉,我无法直接帮助您解决该问题。根据您提供的错误信息,看起来是模型加载时出现了一些问题。建议您检查以下几个方面来解决该问题:
1. 检查模型文件:确保模型文件完整且没有损坏。您可以尝试重新下载或获取一个可靠的模型文件。
2. 检查模型配置:确保您正在使用正确的模型配置文件。有时候加载模型时可能会使用错误的配置文件导致加载失败。
3. 检查依赖项:确认您的环境中安装了所需的依赖项和库。有时候加载模型需要特定版本的库或依赖项才能正常工作。
如果以上步骤都没有解决问题,我建议您参考相关文档或在相关的开发者社区中寻求帮助,以获得更专业的支持。
相关问题
backbone.conv.0.weight", "backbone.conv.0.bias
The "backbone.conv.0.weight" and "backbone.conv.0.bias" are parameters of a neural network's convolutional layer.
The "backbone" refers to the part of the neural network that processes the input data, which is typically an image. The "conv" stands for convolutional layer, which applies a set of filters (represented by the weights) to the input image to extract features.
The "0" refers to the index of the first convolutional layer in the backbone. The "weight" parameter is a tensor that contains the values for the weights, while the "bias" parameter is a tensor that contains the values for the biases added to each filter's output.
These parameters are learned during the training process, where the neural network is tuned to minimize the difference between its predicted output and the desired output. The weights and biases of the convolutional layer are updated through a process called backpropagation, which adjusts them to better capture the relevant features in the input data.
import torch import torch.nn as nn import torchvision.models as models class FCNTransformer(nn.Module): def __init__(self, num_classes): super(FCNTransformer, self).__init__() # Load pre-trained V16 model as FCN backbone vgg16 = models.vgg16(pretrained=True) features = list(vgg16.features.children()) self.backbone = nn.Sequential(*features) # FCN layers self.fcn_layers = nn.Sequential( nn.Conv2d(512, 4096, 7), nn.ReLU(inplace=True), nn.Dropout(), nn.Conv2d(4096, 4096, 1), nn.ReLU(inplace=True), nn.Dropout(), nn.Conv2d(4096, num_classes, 1) ) # Transformer layers self.transformer = nn.Transformer( d_model=512, nhead=8, num_encoder_layers=6, num_decoder_layers=6, dim_feedforward=2048, dropout=0.1 ) def forward(self,x): # Backbone feature extraction features = self.backbone(x) # FCN layers fcn_out = self.fcn_layers(features) # Reshaping output for transformer input b, c, h, w = fcn_out.size() fcn_out = fcn_out.squeeze().view(c, b, -1).permute(2, 0, 1) # Reshaping for transformer input # Transformer encoding transformer_out = self.transformer.encode(fcn_out) # Reshaping output for segmentation prediction transformer_out = transformer_out.permute(1, 2, 0).view(b, c, h, w) return transformer_out if __name__ == '__main__': a = torch.randn(1, 3, 512, 512) model = FCNTransformer(num_classes=2) print(model(a).shape) 改进这段代码
这段代码看起来已经很完整了,但有一些改进可以提出。
首先,由于我们只需要使用 VGG16 的特征提取部分作为 FCN 的 backbone,而不需要其完整的网络结构,因此可以使用 `vgg16.features` 而不是 `vgg16` 来加载预训练模型。这样可以减少模型的参数量。
其次,为了更好地利用 GPU 的性能,可以将输入数据和模型移动到 GPU 上进行计算。可以使用 `to()` 方法将数据和模型移动到 GPU 上,如 `model.to(device)` 和 `a.to(device)`,其中 `device` 是你希望在其上运行代码的设备(如 `'cuda'`)。
最后,你可以考虑添加一些必要的注释来解释代码的功能和每个部分的作用。这有助于其他人更好地理解你的代码。
下面是改进后的代码:
```python
import torch
import torch.nn as nn
import torchvision.models as models
class FCNTransformer(nn.Module):
def __init__(self, num_classes):
super(FCNTransformer, self).__init__()
# Load pre-trained V16 model as FCN backbone
vgg16 = models.vgg16(pretrained=True)
features = list(vgg16.features.children())
self.backbone = nn.Sequential(*features)
# FCN layers
self.fcn_layers = nn.Sequential(
nn.Conv2d(512, 4096, 7),
nn.ReLU(inplace=True),
nn.Dropout(),
nn.Conv2d(4096, 4096, 1),
nn.ReLU(inplace=True),
nn.Dropout(),
nn.Conv2d(4096, num_classes, 1)
)
# Transformer layers
self.transformer = nn.Transformer(
d_model=512,
nhead=8,
num_encoder_layers=6,
num_decoder_layers=6,
dim_feedforward=2048,
dropout=0.1
)
def forward(self, x):
# Backbone feature extraction
features = self.backbone(x)
# FCN layers
fcn_out = self.fcn_layers(features)
# Reshaping output for transformer input
b, c, h, w = fcn_out.size()
fcn_out = fcn_out.squeeze().view(c, b, -1).permute(2, 0, 1) # Reshaping for transformer input
# Transformer encoding
transformer_out = self.transformer.encode(fcn_out)
# Reshaping output for segmentation prediction
transformer_out = transformer_out.permute(1, 2, 0).view(b, c, h, w)
return transformer_out
if __name__ == '__main__':
device = torch.device('cuda' if torch.cuda.is_available() else 'cpu')
a = torch.randn(1, 3, 512, 512).to(device)
model = FCNTransformer(num_classes=2).to(device)
print(model(a).shape)
```
这样,你的代码就更完善了,同时也考虑了在 GPU 上进行计算的情况。
阅读全文
相关推荐
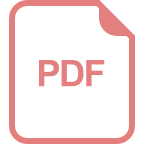
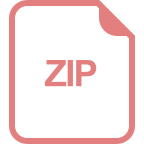
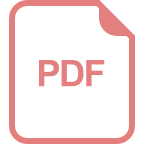













