c++代码编写实现输出素数n(如n=47)的所有原根
时间: 2024-12-19 12:18:10 浏览: 7
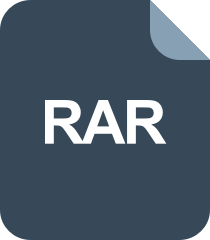
利用c++语言来输出1-100之间的所有素数.rar
在C++中,我们可以编写一个函数来找出给定数n的所有原根。原根是指能够整除所有小于n且与n互质的正整数的最小正整数。以下是使用埃拉托斯特尼筛法(Sieve of Eratosthenes)以及欧几里得算法来查找n的所有原根的基本步骤:
```cpp
#include <iostream>
#include <vector>
using namespace std;
// Function to find the gcd (greatest common divisor)
int gcd(int a, int b) {
if (b == 0)
return a;
return gcd(b, a % b);
}
// Function to check if x is a primitive root modulo n
bool isPrimitiveRoot(int n, int x) {
for (int i = 2; i * i <= n - 1; ++i) {
if ((x % i == 0) && (gcd(x, n) != 1))
return false;
}
if (x == 1 || x == n - 1)
return false;
return true;
}
// Function to print all primitive roots of n
void printPrimitiveRoots(int n) {
vector<int> primes; // To store prime factors of n
for (int i = 2; i * i <= n; ++i) {
while (n % i == 0) {
primes.push_back(i);
n /= i;
}
}
if (n > 1)
primes.push_back(n); // If n is a prime number
for (int x : primes) {
if (isPrimitiveRoot(n, x))
cout << x << " ";
}
}
int main() {
int n = 47;
cout << "Prime factors of " << n << ": ";
printPrimitiveRoots(n);
return 0;
}
```
在这个程序中,我们首先找到n的质因数,然后对每个质因数x,检查它是否是n的一个原始根。如果是,则打印出来。
阅读全文
相关推荐
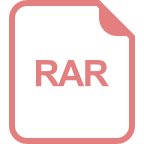
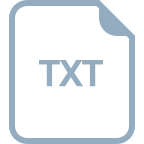














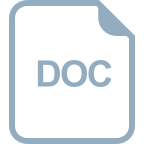
