其余的代码处理输入,构建邻接表,初始化等的具体C++代码
时间: 2024-10-06 10:05:55 浏览: 15
首先,我们需要创建一个Node结构来表示图中的节点,并添加一些辅助函数来处理输入、构建邻接列表和初始化。下面是一个简单的C++代码片段展示如何完成这些任务:
```cpp
#include <iostream>
#include <vector>
#include <string>
using namespace std;
// 定义节点结构
struct Node {
int id;
vector<Node*> neighbors;
bool edge_color; // 边的颜色,白色(true)或黑色(false)
Node(int id) : id(id), edge_color(true) {}
};
// 从输入读取节点和边信息
void read_input(const string& filename, vector<Node>& nodes, vector<pair<int, int>>& edges) {
ifstream fin(filename);
if (!fin.is_open()) {
cerr << "Error opening file!" << endl;
exit(1);
}
int n;
fin >> n;
nodes.resize(n + 1); // 加1以包含根节点
for (int i = 0; i < n - 1; ++i) {
int u, v;
fin >> u >> v;
edges.push_back({u, v});
nodes[u].neighbors.push_back(&nodes[v]);
nodes[v].neighbors.push_back(&nodes[u]); // 添加双向边
}
fin.close();
}
// 构建邻接表
void build_adjacency_list(vector<Node>& nodes) {
for (auto& edge : edges) {
nodes[edge.first].edge_color = true; // 初始设置所有边为白色
}
}
// 主函数
int main() {
string input_filename = "colour.in";
string output_filename = "colour.out";
vector<Node> nodes;
vector<pair<int, int>> edges;
read_input(input_filename, nodes, edges);
build_adjacency_list(nodes);
// ... (处理其他操作)
return 0;
}
// 相关操作函数在这里可以继续添加,如上面提到的changePathColor和countBlackEdges
```
这部分代码首先定义了Node结构,然后定义了一个`read_input`函数来读取输入文件,生成节点和边的列表。接着,`build_adjacency_list`函数用于构建邻接列表并将所有边设为白色。主函数里调用了这两个函数,并开始处理后续的查询操作。
注意这只是一个基本框架,实际操作函数(如`changePathColor`和`countBlackEdges`)需要根据题目需求进一步完善。如果需要处理大规模的数据,可能还需要考虑内存管理和性能优化。
阅读全文
相关推荐
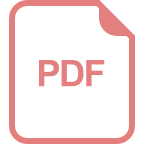
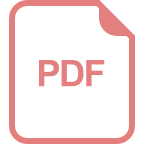
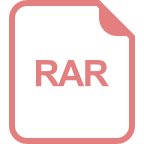
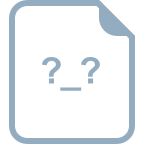
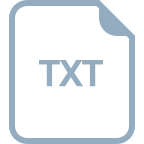
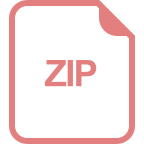
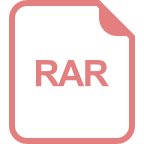
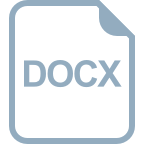
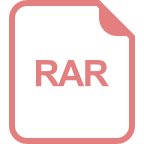
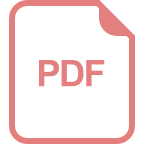
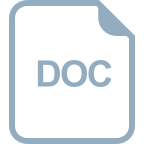
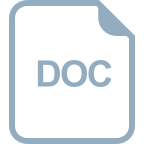
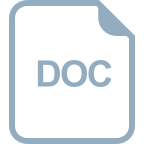
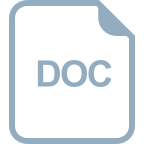
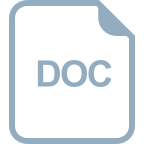



