python hough
时间: 2023-10-11 21:06:37 浏览: 48
Python中的Hough变换是一种常用于图像处理的技术,主要用于检测直线以及其他形状(如矩阵、圆或椭圆)的方法。Hough变换的原理可以简述为以下三句话:首先,将图像空间中的每个点映射到参数空间中,构建一个参数空间的累加器。然后,通过对参数空间中的累加器进行计数,找出累加器值较高的参数对应的直线或形状。最后,将参数空间中的结果反映射回图像空间,得到检测到的直线或形状的位置和属性。
在Python中实现Hough变换的步骤可以大致分为以下几个:
1. 对图像进行预处理,例如灰度化、边缘检测等。
2. 构建Hough空间,并初始化累加器。
3. 对每个边缘点进行遍历,将其映射到Hough空间中,并将相应累加器计数加一。
4. 扫描累加器,找出累加器值较高的参数对应的直线或形状。
5. 反映射结果到图像空间,得到检测到的直线或形状。
以下是使用Python实现Hough变换的示例代码:
```python
import cv2
import numpy as np
# 1. 图像预处理
image = cv2.imread("image.jpg")
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
edges = cv2.Canny(gray, 50, 150)
# 2. 构建Hough空间并初始化累加器
height, width = edges.shape[:2]
rho_max = int(np.sqrt(height**2 + width**2))
theta_max = 180
accumulator = np.zeros((rho_max, theta_max), dtype=np.uint8)
# 3. 边缘点映射到Hough空间并累加器计数
for y in range(height):
for x in range(width):
if edges[y, x] != 0:
for theta in range(theta_max):
rho = int(x * np.cos(np.deg2rad(theta)) + y * np.sin(np.deg2rad(theta)))
accumulator[rho, theta] += 1
# 4. 扫描累加器,找出累加器值较高的参数对应的直线
threshold = 100 # 阈值,用于筛选出高强度的直线
lines = []
for rho in range(rho_max):
for theta in range(theta_max):
if accumulator[rho, theta] > threshold:
a = np.cos(np.deg2rad(theta))
b = np.sin(np.deg2rad(theta))
x0 = a * rho
y0 = b * rho
x1 = int(x0 + 1000 * (-b))
y1 = int(y0 + 1000 * (a))
x2 = int(x0 - 1000 * (-b))
y2 = int(y0 - 1000 * (a))
lines.append(((x1, y1), (x2, y2)))
# 5. 反映射结果到图像空间
result = image.copy()
for line in lines:
cv2.line(result, line[0], line[1], (0, 255, 0), 2)
# 显示结果
cv2.imshow("Result", result)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
相关推荐
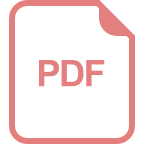
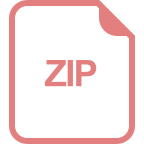
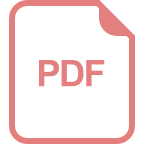














