python opencv 实现hough
时间: 2024-04-08 10:27:04 浏览: 109
Python OpenCV库可以实现Hough变换来检测图像中的直线和圆。下面是使用Python OpenCV实现Hough变换的步骤:
1. 导入必要的库:
```python
import cv2
import numpy as np
```
2. 读取图像并进行预处理:
```python
image = cv2.imread('image.jpg')
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
edges = cv2.Canny(gray, 50, 150)
```
3. 运行Hough变换来检测直线:
```python
lines = cv2.HoughLines(edges, 1, np.pi/180, threshold)
```
其中,`threshold`是用于筛选直线的阈值参数。
4. 绘制检测到的直线:
```python
if lines is not None:
for line in lines:
rho, theta = line[0]
a = np.cos(theta)
b = np.sin(theta)
x0 = a * rho
y0 = b * rho
x1 = int(x0 + 1000 * (-b))
y1 = int(y0 + 1000 * (a))
x2 = int(x0 - 1000 * (-b))
y2 = int(y0 - 1000 * (a))
cv2.line(image, (x1, y1), (x2, y2), (0, 0, 255), 2)
```
5. 运行Hough变换来检测圆:
```python
circles = cv2.HoughCircles(edges, cv2.HOUGH_GRADIENT, dp, minDist, param1=param1, param2=param2, minRadius=minRadius, maxRadius=maxRadius)
```
其中,`dp`是累加器分辨率与图像分辨率的比值,`minDist`是圆心之间的最小距离,`param1`和`param2`是用于筛选圆的阈值参数,`minRadius`和`maxRadius`是圆的最小半径和最大半径。
6. 绘制检测到的圆:
```python
if circles is not None:
circles = np.round(circles[0, :]).astype("int")
for (x, y, r) in circles:
cv2.circle(image, (x, y), r, (0, 255, 0), 2)
```
7. 显示结果:
```python
cv2.imshow("Result", image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
阅读全文
相关推荐
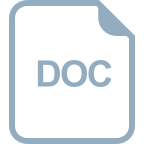
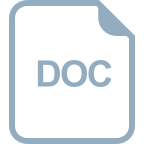
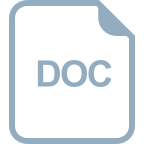



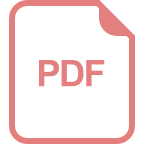
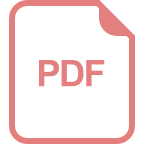
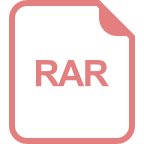
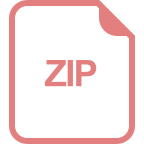
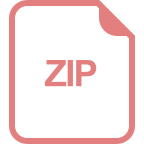
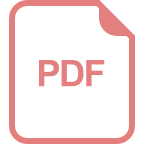
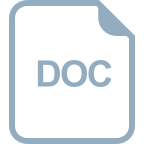





