在c++里如果需要用switch来判断以std::string来分类的情况,应该怎么写
时间: 2024-12-26 13:29:54 浏览: 6
在 C++ 中,由于 `std::string` 类型不是整数或枚举类型,直接无法作为 switch 语句的表达式。不过你可以通过将字符串转换为某种可以用于 switch 的数据类型,或者使用 `if-else if-else` 链接条件来实现类似的功能。以下是两种常见做法:
1. **将字符串转换为整数(仅适用于特定范围内的字符串)**:
如果你的字符串代表的是预定义的整数值,比如 "red", "green", "blue" 对应 0、1、2,你可以创建一个映射表(如 std::map 或 std::unordered_map),然后使用 `stoi()` 函数将字符串转换为整数。
```cpp
#include <string>
#include <map>
std::map<std::string, int> color_map = {{"red", 0}, {"green", 1}, {"blue", 2}};
int classify_color(const std::string& color) {
auto it = color_map.find(color);
if (it != color_map.end()) {
return it->second;
}
// 处理未找到的情况...
}
int main() {
std::string input = "red";
switch (classify_color(input)) {
case 0:
// do something for red
break;
case 1:
// do something for green
break;
case 2:
// do something for blue
break;
default:
// handle unknown color
}
}
```
2. **使用 `if-else if-else` 条件判断**:
如果需要更复杂的匹配规则,可以直接使用 `if-else if-else` 结构。
```cpp
void processColor(const std::string& color) {
if (color == "red") {
// 处理红颜色
} else if (color == "green") {
// 处理绿颜色
} else if (color == "blue") {
// 处理蓝颜色
} else {
// 处理其他颜色,例如未知或错误输入
}
}
int main() {
std::string input = "red";
processColor(input);
}
```
阅读全文
相关推荐
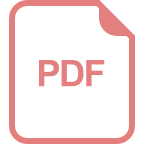
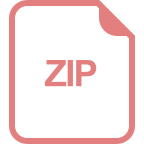
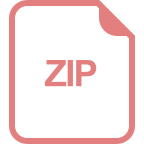


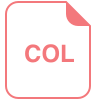
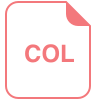
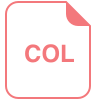
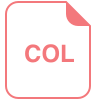
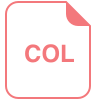
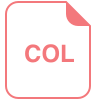
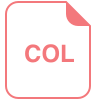
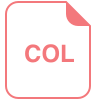
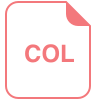





