用js的画布写一个循环烟花特效
时间: 2023-08-19 16:04:49 浏览: 102
当然可以!下面是一个使用 HTML5 的 Canvas 元素和 JavaScript 来实现循环烟花特效的示例代码:
```html
<!DOCTYPE html>
<html>
<head>
<style>
canvas {
background-color: black;
}
</style>
</head>
<body>
<canvas id="myCanvas"></canvas>
<script>
// 获取画布元素
const canvas = document.getElementById("myCanvas");
const ctx = canvas.getContext("2d");
// 设置画布宽高
canvas.width = window.innerWidth;
canvas.height = window.innerHeight;
// 定义烟花数组
const fireworks = [];
// 定义烟花类
class Firework {
constructor(x, y) {
this.x = x;
this.y = y;
this.vx = Math.random() * 2 - 1; // 随机水平速度
this.vy = Math.random() * -8 - 3; // 随机垂直速度,使其向上移动
this.alpha = 1; // 初始透明度
this.color = getRandomColor();
this.exploded = false; // 是否已经爆炸
this.particles = []; // 烟花爆炸后的粒子数组
}
update() {
if (!this.exploded) {
// 更新烟花位置和速度
this.x += this.vx;
this.y += this.vy;
this.vy += 0.1; // 增加垂直速度,使其向下加速
// 绘制烟花
ctx.globalAlpha = this.alpha;
ctx.fillStyle = this.color;
ctx.beginPath();
ctx.arc(this.x, this.y, 3, 0, Math.PI * 2);
ctx.closePath();
ctx.fill();
// 当烟花垂直速度为正值时,表示烟花上升到顶点,此时触发爆炸
if (this.vy >= 0) {
this.explode();
}
} else {
// 更新烟花爆炸后的粒子位置和速度
for (let i = 0; i < this.particles.length; i++) {
const particle = this.particles[i];
particle.x += particle.vx;
particle.y += particle.vy;
particle.vy += 0.1; // 增加垂直速度,使其向下加速
particle.alpha -= 0.02; // 减小透明度
// 绘制烟花粒子
ctx.globalAlpha = particle.alpha;
ctx.fillStyle = particle.color;
ctx.beginPath();
ctx.arc(particle.x, particle.y, 2, 0, Math.PI * 2);
ctx.closePath();
ctx.fill();
// 移除透明度小于0的粒子
if (particle.alpha <= 0) {
this.particles.splice(i, 1);
i--;
}
}
}
}
explode() {
this.exploded = true;
// 生成烟花爆炸后的粒子
for (let i = 0; i < 50; i++) {
const particle = new Particle(this.x, this.y);
this.particles.push(particle);
}
}
}
// 定义粒子类
class Particle {
constructor(x, y) {
this.x = x;
this.y = y;
this.vx = Math.random() * 6 - 3; // 随机水平速度
this.vy = Math.random() * 6 - 3; // 随机垂直速度
this.alpha = 1; // 初始透明度
this.color = getRandomColor();
}
}
// 生成随机颜色
function getRandomColor() {
const letters = "0123456789ABCDEF";
let color = "#";
for (let i = 0; i < 6; i++) {
color += letters[Math.floor(Math.random() * 16)];
}
return color;
}
// 绘制循环烟花特效
function drawFireworks() {
ctx.clearRect(0, 0, canvas.width, canvas.height);
// 更新和绘制烟花
for (let i = 0; i < fireworks.length; i++) {
const firework = fireworks[i];
firework.update();
// 移除已经爆炸完全的烟花
if (firework.exploded && firework.particles.length === 0) {
fireworks.splice(i, 1);
i--;
}
}
// 每隔一段时间生成一个新的烟花
if (Math.random() < 0.05) {
const firework = new Firework(Math.random() * canvas.width, canvas.height);
fireworks.push(firework);
}
requestAnimationFrame(drawFireworks);
}
// 启动循环烟花特效
drawFireworks();
</script>
</body>
</html>
```
将以上代码保存为一个 HTML 文件,并在浏览器中打开,就可以看到循环烟花特效了。希望能满足你的需求!
阅读全文
相关推荐
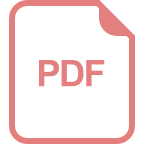

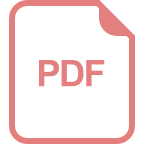
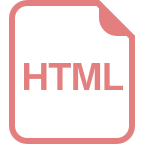
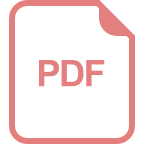
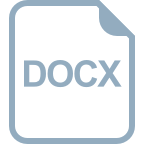
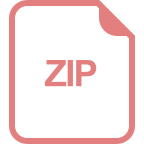
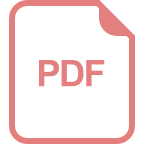
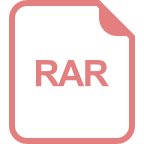
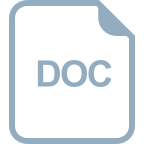
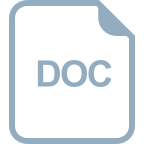
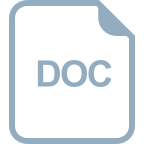
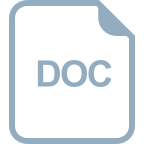
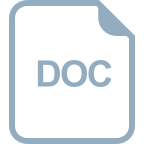


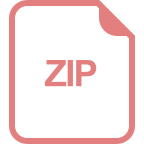