帮我用JavaScript写一个烟花特效
时间: 2023-05-20 17:07:00 浏览: 95
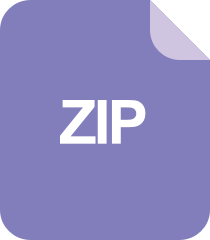
跨年烟花代码JavaScript烟花特效.zip

好的,以下是一个简单的JavaScript烟花特效代码:
```javascript
// 获取画布和上下文
var canvas = document.getElementById("canvas");
var ctx = canvas.getContext("2d");
// 设置画布大小
canvas.width = window.innerWidth;
canvas.height = window.innerHeight;
// 定义烟花数组
var fireworks = [];
// 定义烟花类
function Firework() {
// 烟花的起点坐标
this.x = Math.random() * canvas.width;
this.y = canvas.height;
// 烟花的目标坐标
this.targetX = Math.random() * canvas.width;
this.targetY = Math.random() * canvas.height / 2;
// 烟花的半径和颜色
this.radius = 2;
this.color = "rgb(" + Math.floor(Math.random() * 255) + "," + Math.floor(Math.random() * 255) + "," + Math.floor(Math.random() * 255) + ")";
// 烟花的速度和加速度
this.speed = 5;
this.acceleration = 1.05;
// 烟花是否已经爆炸
this.exploded = false;
// 烟花的粒子数组
this.particles = [];
// 更新烟花的位置
this.update = function() {
// 如果烟花还没有爆炸
if (!this.exploded) {
// 计算烟花的速度和方向
var dx = this.targetX - this.x;
var dy = this.targetY - this.y;
var distance = Math.sqrt(dx * dx + dy * dy);
var vx = dx / distance * this.speed;
var vy = dy / distance * this.speed;
// 更新烟花的位置和速度
this.x += vx;
this.y += vy;
this.speed *= this.acceleration;
// 如果烟花到达目标位置,就让它爆炸
if (distance < 10) {
this.exploded = true;
this.explode();
}
} else {
// 更新烟花的粒子位置
for (var i = 0; i < this.particles.length; i++) {
this.particles[i].update();
}
}
};
// 绘制烟花
this.draw = function() {
if (!this.exploded) {
ctx.beginPath();
ctx.arc(this.x, this.y, this.radius, 0, Math.PI * 2);
ctx.fillStyle = this.color;
ctx.fill();
} else {
for (var i = 0; i < this.particles.length; i++) {
this.particles[i].draw();
}
}
};
// 烟花爆炸
this.explode = function() {
// 创建粒子
for (var i = 0; i < 50; i++) {
var particle = new Particle(this.x, this.y, this.color);
this.particles.push(particle);
}
};
}
// 定义粒子类
function Particle(x, y, color) {
// 粒子的起点坐标
this.x = x;
this.y = y;
// 粒子的半径和颜色
this.radius = 2;
this.color = color;
// 粒子的速度和加速度
this.speed = Math.random() * 5 + 1;
this.acceleration = 1.05;
// 粒子的方向
this.direction = Math.random() * Math.PI * 2;
// 更新粒子的位置
this.update = function() {
// 计算粒子的速度和方向
var vx = Math.cos(this.direction) * this.speed;
var vy = Math.sin(this.direction) * this.speed;
// 更新粒子的位置和速度
this.x += vx;
this.y += vy;
this.speed *= this.acceleration;
this.radius *= 0.96;
};
// 绘制粒子
this.draw = function() {
ctx.beginPath();
ctx.arc(this.x, this.y, this.radius, 0, Math.PI * 2);
ctx.fillStyle = this.color;
ctx.fill();
};
}
// 创建烟花
function createFirework() {
var firework = new Firework();
fireworks.push(firework);
}
// 更新画布
function update() {
// 清空画布
ctx.clearRect(0, 0, canvas.width, canvas.height);
// 更新烟花
for (var i = 0; i < fireworks.length; i++) {
fireworks[i].update();
fireworks[i].draw();
if (fireworks[i].exploded && fireworks[i].particles.length == 0) {
fireworks.splice(i, 1);
}
}
// 创建新的烟花
if (Math.random() < 0.1) {
createFirework();
}
// 重复更新画布
requestAnimationFrame(update);
}
// 开始更新画布
update();
```
你可以将这段代码复制到一个 HTML 文件中,然后在浏览器中打开这个文件,就可以看到烟花特效了。
阅读全文
相关推荐
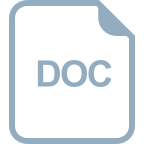
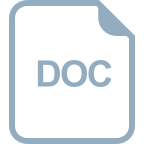
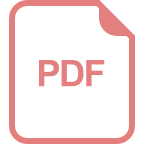
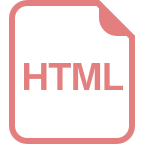
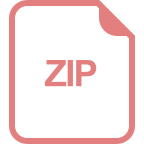
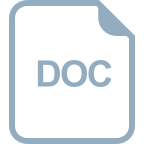
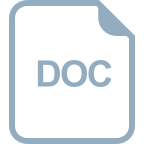
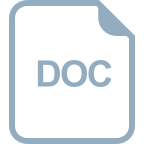
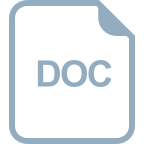






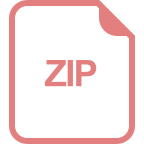
