用html写一个烟花特效
时间: 2023-05-19 17:06:44 浏览: 144
可以使用HTML5和JavaScript来实现烟花特效。以下是一个简单的示例代码:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>烟花特效</title>
<style>
canvas {
position: absolute;
top: 0;
left: 0;
z-index: -1;
}
</style>
</head>
<body>
<canvas id="canvas"></canvas>
<script>
var canvas = document.getElementById("canvas");
canvas.width = window.innerWidth;
canvas.height = window.innerHeight;
var ctx = canvas.getContext("2d");
function random(min, max) {
return Math.random() * (max - min) + min;
}
function Firework() {
this.x = random(0, canvas.width);
this.y = canvas.height;
this.vx = random(-3, 3);
this.vy = random(-20, -10);
this.color = "rgb(" + Math.floor(random(0, 255)) + "," + Math.floor(random(0, 255)) + "," + Math.floor(random(0, 255)) + ")";
this.radius = random(2, 6);
this.alpha = 1;
this.gravity = 0.2;
this.exploded = false;
this.particles = [];
}
Firework.prototype.update = function() {
if (!this.exploded) {
this.x += this.vx;
this.y += this.vy;
this.vy += this.gravity;
if (this.vy >= 0) {
this.exploded = true;
this.explode();
}
} else {
this.alpha -= 0.02;
if (this.alpha <= 0) {
this.alpha = 0;
}
for (var i = 0; i < this.particles.length; i++) {
this.particles[i].update();
}
}
};
Firework.prototype.explode = function() {
for (var i = 0; i < 50; i++) {
var particle = new Particle(this.x, this.y, this.color);
this.particles.push(particle);
}
};
Firework.prototype.draw = function() {
ctx.beginPath();
ctx.arc(this.x, this.y, this.radius, 0, Math.PI * 2, false);
ctx.fillStyle = this.color;
ctx.globalAlpha = this.alpha;
ctx.fill();
ctx.closePath();
if (this.exploded) {
for (var i = 0; i < this.particles.length; i++) {
this.particles[i].draw();
}
}
};
function Particle(x, y, color) {
this.x = x;
this.y = y;
this.vx = random(-5, 5);
this.vy = random(-5, 5);
this.color = color;
this.radius = random(1, 3);
this.alpha = 1;
this.gravity = 0.1;
}
Particle.prototype.update = function() {
this.x += this.vx;
this.y += this.vy;
this.vy += this.gravity;
this.alpha -= 0.02;
if (this.alpha <= 0) {
this.alpha = 0;
}
};
Particle.prototype.draw = function() {
ctx.beginPath();
ctx.arc(this.x, this.y, this.radius, 0, Math.PI * 2, false);
ctx.fillStyle = this.color;
ctx.globalAlpha = this.alpha;
ctx.fill();
ctx.closePath();
};
var fireworks = [];
function loop() {
ctx.clearRect(0, 0, canvas.width, canvas.height);
for (var i = 0; i < fireworks.length; i++) {
fireworks[i].update();
fireworks[i].draw();
if (fireworks[i].alpha <= 0) {
fireworks.splice(i, 1);
}
}
if (Math.random() < 0.05) {
var firework = new Firework();
fireworks.push(firework);
}
requestAnimationFrame(loop);
}
loop();
</script>
</body>
</html>
```
这段代码会在页面上创建一个全屏的canvas元素,并在其中实现烟花特效。每隔一段时间会随机生成一个烟花,并在烟花爆炸时生成多个粒子,形成烟花效果。
阅读全文
相关推荐

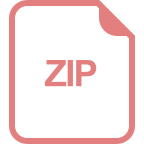












