帮我用JavaScript写一个烟花效果
时间: 2024-05-04 17:19:49 浏览: 75
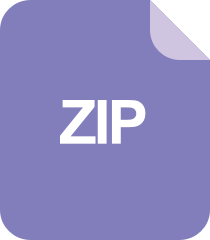
跨年烟花代码-javascript烟花星空魔法师源代码下载
好的,以下是一个简单的烟花效果的JavaScript代码:
```javascript
// 获取画布和上下文
const canvas = document.querySelector('canvas');
const ctx = canvas.getContext('2d');
// 设置画布大小
canvas.width = window.innerWidth;
canvas.height = window.innerHeight;
// 创建烟花粒子类
class Particle {
constructor(x, y, color) {
this.x = x;
this.y = y;
this.color = color;
this.radius = 3;
this.velocity = {
x: Math.random() * 4 - 2,
y: Math.random() * 4 - 2
};
this.alpha = 1;
}
// 更新粒子位置和透明度
update() {
this.x += this.velocity.x;
this.y += this.velocity.y;
this.alpha -= 0.02;
}
// 绘制粒子
draw() {
ctx.beginPath();
ctx.arc(this.x, this.y, this.radius, 0, Math.PI * 2);
ctx.fillStyle = this.color;
ctx.globalAlpha = this.alpha;
ctx.fill();
ctx.closePath();
}
}
// 创建烟花类
class Firework {
constructor(x, y, color) {
this.x = x;
this.y = y;
this.color = color;
this.particles = [];
for (let i = 0; i < 50; i++) {
this.particles.push(new Particle(this.x, this.y, this.color));
}
}
// 更新烟花和粒子状态
update() {
for (let i = 0; i < this.particles.length; i++) {
this.particles[i].update();
if (this.particles[i].alpha <= 0) {
this.particles.splice(i, 1);
}
}
}
// 绘制烟花和粒子
draw() {
for (let i = 0; i < this.particles.length; i++) {
this.particles[i].draw();
}
}
}
// 存储烟花
const fireworks = [];
// 鼠标点击事件
canvas.addEventListener('click', event => {
const x = event.clientX;
const y = event.clientY;
const colors = ['#ff0000', '#00ff00', '#0000ff', '#ffff00', '#00ffff', '#ff00ff'];
const color = colors[Math.floor(Math.random() * colors.length)];
fireworks.push(new Firework(x, y, color));
});
// 动画循环
function loop() {
// 清除画布
ctx.fillStyle = 'rgba(0, 0, 0, 0.1)';
ctx.fillRect(0, 0, canvas.width, canvas.height);
// 更新和绘制烟花
for (let i = 0; i < fireworks.length; i++) {
fireworks[i].update();
fireworks[i].draw();
}
// 循环动画
requestAnimationFrame(loop);
}
// 启动动画
loop();
```
在 HTML 文件中添加一个 canvas 元素,并引入上面的 JavaScript 代码,就可以看到烟花效果了。
阅读全文
相关推荐
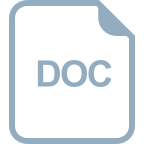
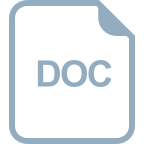

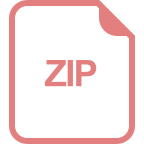







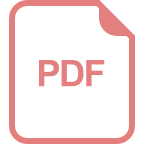
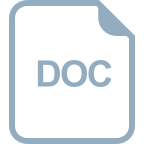
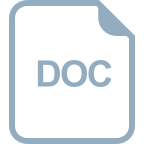



