void GetMemory( char *p ) { p = (char *) malloc( 100 ); } void main() { char *str = NULL; GetMemory( str ); strcpy( str, hello world );printf( str ); }
时间: 2024-04-15 10:27:37 浏览: 166
代码中存在几个问题:
1. 在函数 `GetMemory()` 中,你想要分配内存并将其地址赋给指针 `p`,但是这里的 `p` 是一个局部变量,对其进行赋值不会影响到 `main()` 函数中的指针 `str`。
2. 在 `main()` 函数中,你将 `str` 初始化为 `NULL`,然后将其作为参数传递给 `GetMemory()` 函数。但是由于问题1,`str` 的值并没有被修改为新分配的内存地址,因此在之后的 `strcpy()` 和 `printf()` 中使用 `str` 会导致未定义的行为。
3. 在 `strcpy()` 中,字符串应该用双引号括起来,即 `"hello world"`。
为了修复这些问题,你可以修改代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
void GetMemory(char **p) {
*p = (char *) malloc(100);
}
int main() {
char *str = NULL;
GetMemory(&str);
strcpy(str, "hello world");
printf("%s\n", str);
free(str); // 释放内存
return 0;
}
```
这样修改后,`GetMemory()` 函数使用了一个指向指针的指针作为参数,通过修改指针的值来使其指向新分配的内存。在 `main()` 函数中传递 `&str` 来获取指向 `str` 的指针的地址。另外,`strcpy()` 中的字符串使用双引号括起来,并且在 `printf()` 中使用 `%s` 来打印字符串。最后,记得在不再使用内存时调用 `free()` 来释放它。
阅读全文
相关推荐





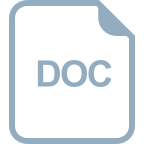






