AttributeError: 'NoneType' object has no attribute 'status_code'
时间: 2023-12-06 09:03:47 浏览: 48
这个错误通常是因为你尝试访问一个None对象的属性或方法,而None对象没有该属性或方法。通常情况下,这是因为你的代码中出现了错误,导致你的变量被赋值为None,而你尝试在该变量上调用方法或属性。例如,在使用requests库时,如果你的请求返回了None,那么你尝试访问该请求的状态码属性时就会出现这个错误。
以下是一个例子,演示了如何在requests库中出现这个错误:
```python
import requests
response = requests.get('https://www.google.com')
print(response.status_code) # 正常输出200
response = requests.get('https://www.nonexistentwebsite.com')
print(response.status_code) # 报错:AttributeError: 'NoneType' object has no attribute 'status_code'
```
在第二个请求中,由于网站不存在,requests库返回了None,而你尝试在该None对象上访问状态码属性,因此出现了这个错误。
相关问题
AttributeError: 'NoneType' object has no attribute 'python_exit_status怎么解决
AttributeError: 'NoneType' object has no attribute 'python_exit_status'是一个常见的错误,通常是由于在程序中使用了未初始化的变量或对象。解决此问题的方法有以下几种:
1.检查代码中是否存在未初始化的变量或对象,如果存在,则需要对其进行初始化。
2.检查代码中是否存在语法错误或拼写错误,这些错误可能会导致程序无法正常运行。
3.使用try-except语句捕获异常并进行处理,例如:
```python
try:
# your code here
except AttributeError:
# handle the exception here
```
4.检查代码中是否存在循环引用或内存泄漏等问题,这些问题可能会导致程序出现异常。
AttributeError: 'NoneType' object has no attribute 'get_input_layers'
针对错误"AttributeError: 'NoneType' object has no attribute 'find_all'",通常是因为代码中的某个变量为None,而None没有find_all属性,因此会报错。解决方法是在使用该变量之前,先判断该变量是否为None,如果是则不执行相关操作。
针对错误"AttributeError: 'NoneType' object has no attribute 'get_input_layers'",同样是因为代码中的某个变量为None,而None没有get_input_layers属性,因此会报错。解决方法同上,需要在使用该变量之前,先判断该变量是否为None,如果是则不执行相关操作。
以下是一个示例代码,用于演示如何避免这两种错误:
```python
import requests
from bs4 import BeautifulSoup
url = 'https://www.example.com'
response = requests.get(url)
if response.status_code == 200:
soup = BeautifulSoup(response.text, 'html.parser')
content = soup.find_all('div', class_='content')
if content is not None:
for item in content:
print(item.text)
else:
print('请求失败')
```
相关推荐
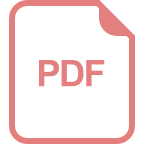
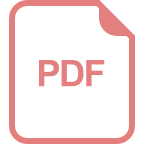
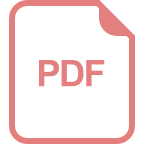










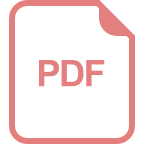
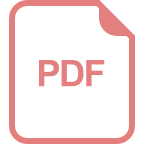
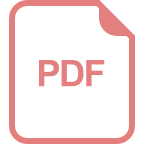