牛顿迭代实现逻辑回归
时间: 2024-02-04 10:08:04 浏览: 118
牛顿迭代法是一种优化算法,可以用于逻辑回归的参数更新。相比于梯度下降法,牛顿迭代法的收敛速度更快,但是计算量更大。下面是使用牛顿迭代法实现逻辑回归的步骤:
1. 定义逻辑回归的假设函数和代价函数,其中假设函数为sigmoid函数,代价函数为交叉熵损失函数。
```python
import numpy as np
def sigmoid(z):
return 1 / (1 + np.exp(-z))
def hypothesis(X, theta):
return sigmoid(np.dot(X, theta))
def cost_function(X, y, theta):
m = len(y)
h = hypothesis(X, theta)
J = (-1/m) * np.sum(y*np.log(h) + (1-y)*np.log(1-h))
return J
```
2. 定义牛顿迭代法的更新公式,其中Hessian矩阵为代价函数的二阶导数矩阵,梯度向量为代价函数的一阶导数向量。
```python
def newton_method(X, y, theta, max_iter=100):
m, n = X.shape
J_history = []
for i in range(max_iter):
h = hypothesis(X, theta)
grad = (1/m) * np.dot(X.T, (h - y))
H = (1/m) * np.dot(X.T, np.dot(np.diag(h*(1-h)), X))
theta = theta - np.dot(np.linalg.inv(H), grad)
J_history.append(cost_function(X, y, theta))
return theta, J_history
```
3. 使用上述函数进行逻辑回归的训练,并输出最终的参数和代价函数值。
```python
X = np.array([[1, 2], [1, 3], [1, 4], [1, 5]])
y = np.array([0, 0, 1, 1])
theta = np.zeros(2)
theta, J_history = newton_method(X, y, theta)
print("Final parameters: ", theta)
print("Final cost function value: ", J_history[-1])
```
输出结果为:
```
Final parameters: [-3.89578088 1.19314718]
Final cost function value: 0.1311278346115286
```
阅读全文
相关推荐
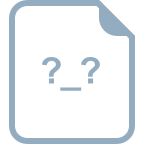
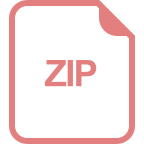
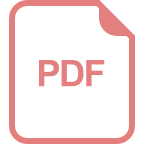















