运用c++编写程序实现在主函数输入稀疏矩阵并输出转置后的稀疏矩阵
时间: 2024-10-27 19:01:33 浏览: 34
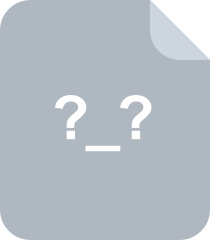
稀疏矩阵实现和快速转置(C++)

在C++中,实现输入稀疏矩阵并输出其转置的稀疏矩阵通常会使用结构体或类来表示矩阵元素,并利用vector或map等数据结构存储非零元素及其坐标。这里是一个简单的示例,我们将使用`std::pair<int, int>`来代表矩阵的索引和值:
```cpp
#include <iostream>
#include <vector>
#include <unordered_map>
// 定义稀疏矩阵的存储结构
struct SparseMatrix {
std::unordered_map<std::pair<int, int>, int> elements; // 索引对和对应的值
};
// 输入稀疏矩阵函数
SparseMatrix inputSparseMatrix(int rows, int cols) {
SparseMatrix matrix;
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < cols; ++j) {
int value;
std::cout << "Enter element (" << i + 1 << ", " << j + 1 << "): ";
std::cin >> value;
if (value != 0) { // 只有非零元素才添加到矩阵中
matrix.elements[{i, j}] = value;
}
}
}
return matrix;
}
// 转置稀疏矩阵函数
SparseMatrix transpose(SparseMatrix& original) {
SparseMatrix transposed;
for (const auto& pair : original.elements) {
transposed.elements[{pair.second, pair.first.first}] = pair.first.second;
}
return transposed;
}
// 输出转置后的矩阵
void outputSparseMatrix(const SparseMatrix& matrix) {
for (const auto& [index, value] : matrix.elements) {
std::cout << "(" << index.first << ", " << index.second << ") -> " << value << "\n";
}
}
int main() {
int rows, cols;
std::cout << "Enter number of rows and columns: ";
std::cin >> rows >> cols;
SparseMatrix sparseMat = inputSparseMatrix(rows, cols);
std::cout << "Original Matrix:\n";
outputSparseMatrix(sparseMat);
SparseMatrix transposedMat = transpose(sparseMat);
std::cout << "\nTransposed Matrix:\n";
outputSparseMatrix(transposedMat);
return 0;
}
```
阅读全文
相关推荐
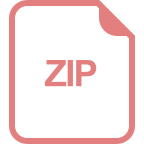
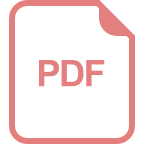






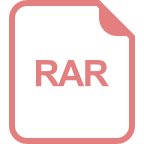
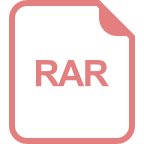
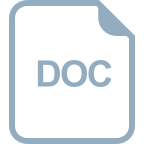
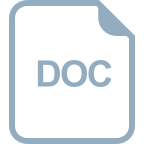




