二叉树的链式存储代码c++
时间: 2024-09-15 19:01:34 浏览: 60
在C++中,我们可以使用结构体或类来表示二叉树的节点,并通过链表的方式存储每个节点的左子节点和右子节点。以下是一个简单的二叉树节点的链式存储结构以及基本的操作(如插入、查找等)的示例:
```cpp
// 定义二叉树节点
class TreeNode {
public:
int val; // 节点值
TreeNode* left; // 左子节点指针
TreeNode* right; // 右子节点指针
// 构造函数
TreeNode(int val = 0, TreeNode* left = nullptr, TreeNode* right = nullptr) :
val(val), left(left), right(right) {}
};
// 插入节点到二叉搜索树
TreeNode* insertIntoBST(TreeNode* root, int val) {
if (root == nullptr) return new TreeNode(val);
if (val < root->val)
root->left = insertIntoBST(root->left, val);
else
root->right = insertIntoBST(root->right, val);
return root;
}
// 查找节点
TreeNode* searchBST(TreeNode* root, int val) {
while (root != nullptr) {
if (root->val == val)
return root;
else if (val < root->val)
root = root->left;
else
root = root->right;
}
return nullptr;
}
// 示例
int main() {
// 创建一个空树
TreeNode* root = nullptr;
// 插入一些节点
root = insertIntoBST(root, 50);
insertIntoBST(root, 30);
insertIntoBST(root, 20);
insertIntoBST(root, 40);
insertIntoBST(root, 70);
insertIntoBST(root, 60);
// 打印整个二叉搜索树(仅做展示,实际应用中可能需要递归遍历)
// ...
return 0;
}
```
阅读全文
相关推荐
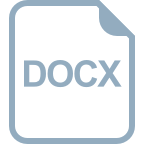
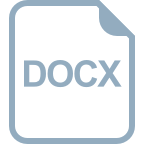
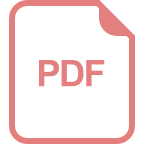
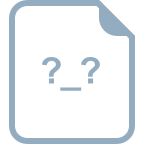



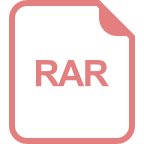
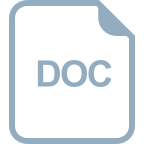








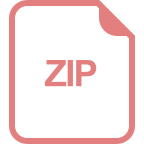