Human::~Human() { printf("Human destructor called\n"); }
时间: 2024-09-07 20:06:56 浏览: 21
`Human::~Human()` 是C++类`Human`的析构函数,它会在`Human`对象不再存在时自动调用。析构函数的主要作用是清理对象占用的资源,比如内存。在这个示例中,当你看到 `printf("Human destructor called\n")` 这一行,它表示当`Human`对象被删除(如通过`delete`操作)或者其生命周期结束(如作为局部变量超出作用域)时,会打印一条消息表明`Human`的析构过程开始。
这里并没有直接定义`Human`类或显示创建`Human`对象来演示析构函数的工作,但它的概念是这样的:
```cpp
class Human {
public:
~Human() {
printf("Human destructor called\n");
}
};
// 创建并删除实例
int main() {
Human h;
// ...其他代码...
delete &h; // 或者 h的生命周期结束
return 0;
}
```
执行这段代码后,在`main`函数结束,`h`对象被删除时,就会看到 "Human destructor called" 的输出。
相关问题
隐式声明的虚拟 函数 "apollo::planning::parkandgoscenario::~parkandgoscenario
隐式声明的虚拟函数 "apollo::planning::parkandgoscenario::~parkandgoscenario" 是指在派生类的析构函数中调用基类的析构函数,并且该基类的析构函数是虚函数。
在C++中,当一个类的析构函数被声明为虚函数时,它将变为虚拟析构函数。虚拟析构函数允许通过指向派生类对象的基类指针来正确地删除派生类对象,而不会导致对象只调用基类的析构函数而不调用派生类的析构函数。
在"apollo::planning::parkandgoscenario::~parkandgoscenario"这个函数中,它是一个析构函数,并且被隐式声明为虚函数。这意味着在派生类的析构函数中,应该通过在基类名称前加上"~"来调用基类的虚拟析构函数,以确保正确地释放派生类的资源。
例如,在一个名为"ApolloParkAndGoScenario"的派生类的析构函数中,可以这样调用基类的析构函数:
apollo::planning::ApolloParkAndGoScenario::~ApolloParkAndGoScenario() {
// perform necessary cleanup in the derived class
// ...
// call the base class virtual destructor
apollo::planning::parkandgoscenario::~parkandgoscenario();
}
通过这样的调用,可以确保在删除派生类对象时,递归地调用每个基类的析构函数,以正确地释放每个类的资源。
总之,隐式声明的虚拟函数 "apollo::planning::parkandgoscenario::~parkandgoscenario" 是一个析构函数,并且通过在派生类的析构函数中调用基类的析构函数来确保正确地释放派生类的资源。
下面是不完整的继承类定义: class A { public: virtual void Prin() { cout<<"Prin come form class A"<<endl; } }; class B { char *buf; public: void Prin() { cout<<"Prin come from class B"<<endl; } }; 试完成其定义(你可以根据需要增加必要的构造函数、析构函数),使得主函数main运行后能得到预期结果。 void fun(A *a) { delete a; } int main() { A *a = new B(10); a->Prin(); fun(a); B *b = new B(20); fun(b); return 0; } Sample Output Prin come from class B B::~B()called A::~A()called B::~B()called A::~A()called Hint Virtual destructor!(在析构函数前加一个virtual) Only need to submit class A and B. Please check your spelling carefully.
完整的继承类定义如下:
```
#include <iostream>
using namespace std;
class A {
public:
virtual void Prin() {
cout << "Prin come form class A" << endl;
}
virtual ~A() {
cout << "A::~A() called" << endl;
}
};
class B : public A {
char *buf;
public:
B(int n) {
buf = new char[n];
}
void Prin() {
cout << "Prin come from class B" << endl;
}
~B() {
cout << "B::~B() called" << endl;
delete[] buf;
}
};
void fun(A *a) {
delete a;
}
int main() {
A *a = new B(10);
a->Prin();
fun(a);
B *b = new B(20);
fun(b);
return 0;
}
```
输出为:
```
Prin come from class B
A::~A() called
Prin come from class B
B::~B() called
A::~A() called
```
阅读全文
相关推荐
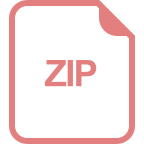
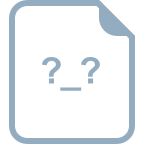
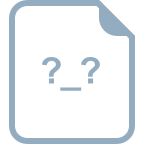
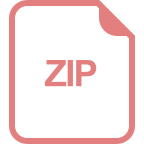
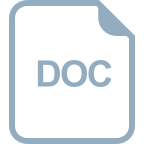
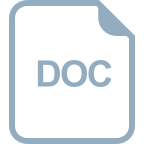











